Cracking the Code: 50 Professional Interview Questions and Answers for Wipro's Software Development Role
- Author
- Feb 11
- 9 min read
When it comes to preparing for a software development interview, especially at a reputable company like Wipro, the stakes can be quite high. Whether you are an entry-level coder or an experienced software engineer, having a well-equipped toolkit of interview questions and effective answers can be your biggest asset.
This blog aims to provide you with fifty common interview questions and well-articulated answers tailored specifically for a software development role at Wipro. This comprehensive collection will not only help you prepare mentally but will also give you insight into what the interviewers are looking for.
Understanding Wipro's Expectations
Wipro is a global leader in technology services and consulting, and they are known for their rigorous recruitment process, especially in the field of software development. As you navigate through these questions, it's important to remember that they are not merely transactional; rather, they focus on gauging your logic, problem-solving abilities, and cultural fit within the organization.
To ensure you make a lasting impression during your interview, take the time to familiarize yourself with Wipro's core values and mission. This understanding can serve as a beneficial guide as you formulate your answers and present your skills.
Technical Questions
1. What is the difference between an abstract class and an interface in Java?
An abstract class can have both abstract methods (without a body) and concrete methods (with a body). An interface, however, can only have abstract methods (prior to Java 8). This crucial distinction plays a significant role in how you structure your Java applications.
2. Can you explain the concept of inheritance in OOP?
Inheritance allows one class to inherit the methods and fields of another class. This supports reusability and can lead to a more logical and organized code structure, as derived classes can build upon the existing functionality of base classes.
3. What is the purpose of the `final` keyword in Java?
The `final` keyword is used to declare constants, prevent method overriding, and prevent inheritance. It essentially locks down the value or function of the variable, method, or class.
4. Could you explain polymorphism with an example?
Polymorphism allows methods to do different things based on the object it is acting upon. For example, if you have a base class called Shape and derived classes like Circle and Square, if both have a method called Draw(), polymorphism allows calling the Draw() method on an object of either class to yield different results.
5. What is the difference between a stack and a queue?
A stack follows a Last In First Out (LIFO) order, while a queue follows a First In First Out (FIFO) order. This fundamental distinction affects how data is managed and accessed in different applications.
Problem Solving Questions
6. How would you reverse a linked list?
You can reverse a linked list by maintaining three pointers: Previous, Current, and Next. Traverse through the list, and at each node, redirect the current node's next pointer to its previous node.
7. What is the time complexity of searching in a binary search tree?
The time complexity of searching in a binary search tree (BST) is O(h), where h is the height of the tree. In the best case (when the tree is balanced), this is O(log n).
8. Describe how you handle version control in your projects.
Utilizing Git allows for efficient version control and collaboration among team members. I maintain feature branches for new developments and regularly merge them back into the main branch after thorough testing.
9. How can you optimize the performance of a SQL query?
You can optimize SQL queries by creating indexes, avoiding `SELECT *`, using proper joins, and analyzing query execution plans to spot bottlenecks.
10. Explain the concept of Big O notation.
Big O notation is a mathematical representation used to describe the upper limit of an algorithm's runtime or space complexity in terms of input size. It provides a way to compare the efficiency of different algorithms.
Behavioral Questions
11. Tell me about a time you faced a significant challenge in a project.
In my previous project, we were faced with a tight deadline and a critical bug was discovered close to release. I coordinated with the team, segregated tasks based on expertise, and we worked collaboratively to address the issues, successfully meeting our deadline.
12. How do you prioritize tasks when managing a project?
I prioritize tasks based on urgency and impact. I create a to-do list, segmenting tasks into categories such as critical, high, medium, and low priority. This way, I ensure that essential tasks get completed first.
13. Describe a situation in which you had to work in a team.
In a collaborative project, I took the initiative to schedule regular meetings for updates and feedback. This approach facilitated better communication, leading to higher productivity and team cohesiveness.
14. How do you ensure the quality of your code?
I follow best practices like writing readable code, conducting peer code reviews, and implementing unit tests. Automated testing and integration checks further enhance code quality and efficiency in the development lifecycle.
15. What motivates you to do your best work?
I am driven by a passion for solving complex problems and the satisfaction of creating efficient, well-structured code. The prospect of learning new technologies and paradigms further fuels my motivation.
Scenario-Based Questions
16. Imagine you've inherited a poorly written codebase. How would you improve it?
I would begin by thoroughly reviewing the existing code to understand its functionality, identifying the most critical parts that require immediate attention, and refactoring them gradually while adding tests to prevent regression.
17. How would you handle a disagreement with a team member regarding coding standards?
I would initiate an open discussion where we can both express our views. I believe in compromising based on best practices while ensuring that we uphold the project's integrity.
18. Suppose you have to work on a technology stack that you're unfamiliar with. How would you approach it?
I'd dedicate time to research and learn the stack, utilizing online resources such as documentation, tutorials, and forums. I would also actively seek help from colleagues who have expertise in the area.
19. If you're tasked with researching a new technology for future projects, what steps would you take?
I would start by gathering requirements and objectives, conducting a detailed analysis of the technology's benefits and limitations, and presenting my findings to the team for feedback and collaboration on a potential transition.
20. What would you do if you realize you won't meet your project deadlines?
I would promptly communicate with relevant stakeholders, explaining the situation and proposing a revised timeline. Additionally, I would seek assistance from team members to redistribute tasks efficiently.
General Knowledge Questions
21. What is Agile methodology?
Agile methodology is an iterative approach to software development that emphasizes flexibility, customer collaboration, and rapid responses to change. It allows teams to deliver functional software in incremental cycles.
22. Explain the concept of REST in web services.
REST (Representational State Transfer) is an architectural style for designing networked applications. It utilizes standard HTTP methods and focuses on stateless communication, enabling scalability and performance in web services.
23. How does garbage collection work in Java?
Garbage collection in Java is an automatic process focusing on reclaiming memory by eliminating objects that are no longer in use. It involves several algorithms to identify and dispose of unreachable objects, optimizing memory usage.
24. Can you explain the difference between process and thread?
A process is an independent program in execution, while a thread is a smaller unit of a process capable of running concurrently. Threads share resources of their parent process, leading to more efficient use of system resources.
25. What are the advantages of microservices architecture?
Microservices architecture allows for modular development, scalability, and easier deployment. Different services can be developed and maintained independently, facilitating agile development and more manageable codebases.
Coding Questions
26. Write a function to check if a string is a palindrome.
```java
public boolean isPalindrome(String str) {
String reversed = new StringBuilder(str).reverse().toString();
return str.equals(reversed);
}
```
The function checks if the string matches its reversed version, returning `true` for palindromes and `false` otherwise.
27. How would you merge two sorted arrays?
You could use a two-pointer approach, comparing elements in both arrays and adding the smaller element to the result array until you've processed all elements.
28. Write a code snippet to find the factorial of a number using recursion.
```java
public int factorial(int n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
```
This recursive function efficiently calculates the factorial by multiplying the number by the factorial of the previous number.
29. Implement a function to find the maximum element in an array.
```java
public int findMax(int[] arr) {
int max = arr[0];
for (int i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
```
This function iterates through the array, updating the maximum value as it compares each element.
30. How would you sort an array using quicksort?
Quicksort is a divide-and-conquer algorithm that sorts an array by selecting a 'pivot' element, partitioning the other elements into two sub-arrays of lesser and greater values, and then recursively sorting those sub-arrays.
Company Culture Questions
31. Why do you want to work at Wipro?
I am impressed with Wipro's commitment to technological innovation and its diverse working environment, which I believe will provide ample opportunities for growth and the ability to work on impactful projects.
32. How do you fit into Wipro's core values?
I resonate strongly with Wipro's core values of integrity, excellence, and customer-centricity. These values guide my professional conduct and decision-making processes.
33. How would you contribute to team dynamics at Wipro?
I actively promote open communication and collaboration. By encouraging diverse viewpoints and fostering a cohesive team environment, I aim to enhance productivity and creative problem-solving.
34. What are your thoughts on continuous learning?
I believe continuous learning is essential in the ever-evolving field of technology. I regularly engage in professional development through workshops, online courses, and community forums.
35. How do you adapt to changes in the workplace?
I maintain a flexible mindset, view changes as opportunities to grow, and actively seek feedback for improvement. Adaptability is key to thriving in dynamic corporate environments.
Career Development Questions
36. What are your career goals in the next five years?
I aspire to grow my expertise in software development, ideally in a lead role where I can mentor others and make strategic contributions to projects. I aim to broaden my skill set through continual learning and hands-on experience.
37. How do you define success in your career?
Success, for me, involves a combination of personal growth, achieving set goals, and contributing positively to my team and organization. I value knowledge, both in breadth and depth.
38. Describe a mentor who influenced your career.
My mentor emphasized the importance of problem-solving and critical thinking. Their guidance has instilled a strong work ethic and a resolve to pursue excellence in everything I undertake.
39. How do you handle feedback?
I welcome constructive criticism as a tool for growth. I evaluate the feedback objectively, reflect on it, and apply what is necessary to enhance my work and skills.
40. What skills do you want to develop further?
I am keen on enhancing my skills in cloud computing and machine learning. Both areas are increasingly significant in software development and I believe they will broaden my capabilities.
Final Questions
41. How do you stay updated on industry trends?
I regularly read technical blogs, attend webinars, and participate in local tech meetups. This helps me stay informed about new tools, frameworks, and methodologies in the software development landscape.
42. What programming languages are you most comfortable with?
I am most comfortable with Java and Python, having used them extensively for both academic and professional projects. I am also familiar with JavaScript and C#.
43. Describe a project that you are particularly proud of.
I developed a mobile application that solved a specific problem in a community. The project involved extensive research and user testing, and it received great feedback upon launch, significantly benefiting users.
44. What is your approach towards debugging?
I systematically isolate parts of the code to identify the source of errors, using debugging tools and logging to trace issues. I ensure to validate each modification carefully to confirm functionality.
45. How do you balance multiple projects?
I rely on time management tools and techniques. Creating a clear timeline with deadlines, as well as regular check-ins, helps ensure that all projects stay on track and receive appropriate attention.
Conclusion
Preparing for your software development interview at Wipro doesn’t have to be an overwhelming process. By familiarizing yourself with these fifty common interview questions and carefully crafting your answers with confidence, you're setting yourself up for success.
With a well-rounded understanding of necessary technical skills, behavioral aspects, and a conscientious approach to coding challenges, you can impress your interviewers and secure your place in this esteemed organization.
Remember, preparation is key. Good luck!
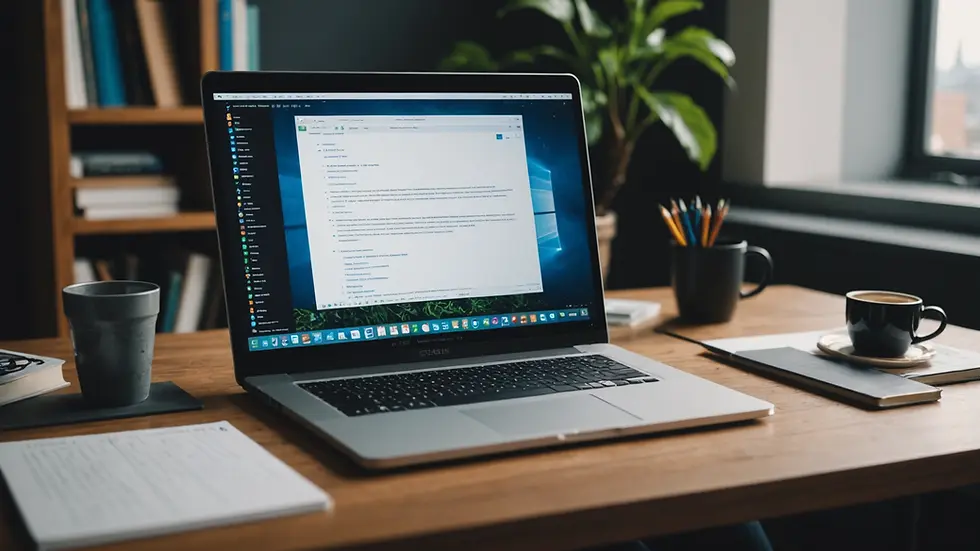
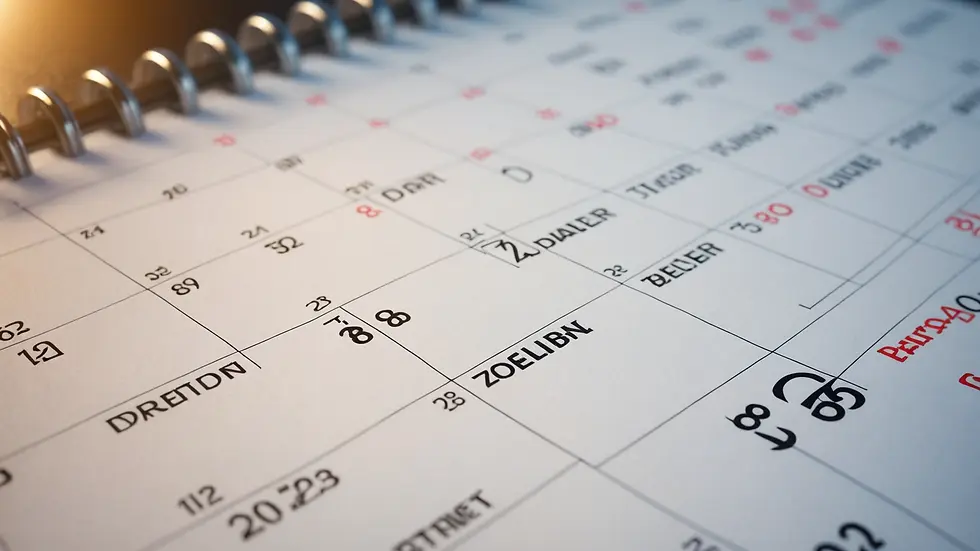
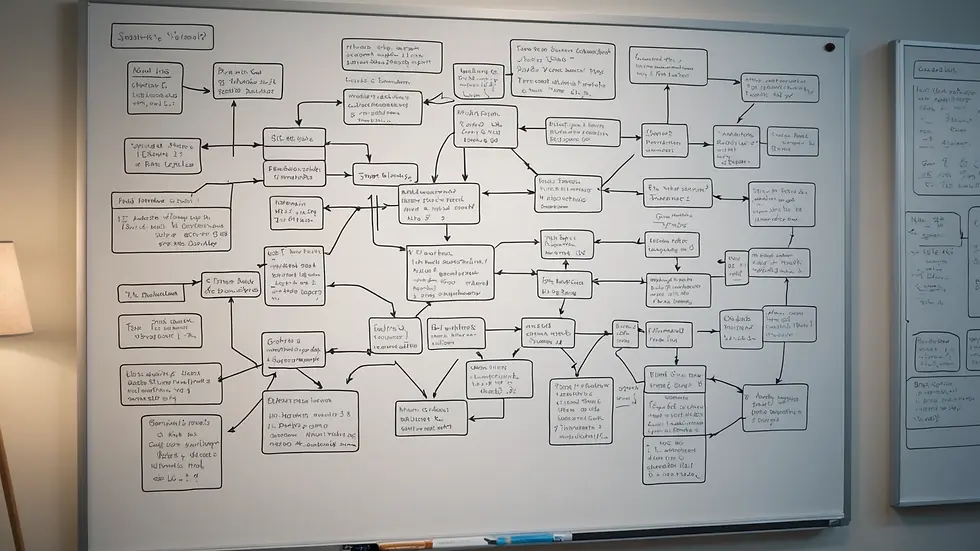