Interview Question and Answers for the role of Backend Developer at Abridge
- Author
- Feb 11
- 9 min read
The path to landing a Backend Developer position is both thrilling and challenging. To succeed in interviews, especially with a forward-thinking company like Abridge that is committed to enhancing healthcare technology, thorough preparation is key. This blog post explores 50 common interview questions, along with practical answers aimed at helping both aspiring and experienced backend developers.
Understanding the Role of a Backend Developer
A backend developer focuses on the server-side of web applications, ensuring everything runs smoothly behind the scenes. This involves database management, server logic, and maintaining effective communication between the client and the server. Proficiency in various programming languages, frameworks, and database technologies is essential.
1. What is backend development?
Backend development is the server-side development of an application that occurs behind the scenes, managing business logic, database interactions, and server communications.
2. What programming languages are you proficient in?
I am proficient in several programming languages such as Java, Python, and Node.js. For example, Java is widely used in enterprise applications, while Python excels in data manipulation and web frameworks like Django.
3. Can you explain the MVC architecture?
MVC stands for Model-View-Controller. In this architecture:
Model deals with data and business logic.
View renders the user interface.
Controller handles input and updates the model.
This separation aids in maintaining organized code.
4. What is RESTful API?
A RESTful API conforms to REST principles, which facilitate communication between different systems using standard HTTP methods like GET, POST, PUT, and DELETE. For instance, a mobile app might communicate with a backend RESTful API to fetch user data.
5. Can you explain what CRUD operations are?
CRUD stands for Create, Read, Update, and Delete—fundamental operations needed to manage data effectively. For example, in a library management system, you would Create a new book record, Read existing records, Update information on a book, or Delete a record when a book is removed from the inventory.
6. How do you handle authentication in backend applications?
Authentication can be implemented using:
Tokens (e.g., JWT, OAuth): Widely used for securing APIs.
Sessions: Often used in traditional web applications to maintain user state.
Each method is selected based on the application's security requirements.
7. What is the difference between SQL and NoSQL databases?
SQL databases, such as MySQL, follow a structured, relational approach requiring predefined schemas, making them suitable for complex queries. On the other hand, NoSQL databases like MongoDB offer flexibility, allowing for unstructured data storage, which is particularly useful for applications that manage large volumes of varied data.
8. Explain what an HTTP status code is.
HTTP status codes inform clients about the outcome of their requests. For example:
200 indicates success.
404 means the requested resource was not found.
These codes guide users and developers in identifying issues quickly.
9. Can you describe how you optimize your backend code?
I optimize backend code by:
Minimizing Database Calls: Reducing the frequency of queries.
Using Caching Strategies: Implementing Redis or Memcached to cache frequently requested data.
Efficient Algorithms: Choosing appropriate algorithms and data structures can enhance overall performance significantly.
10. What is a microservices architecture?
Microservices architecture involves creating software applications as a collection of small, independently deployable services that communicate over APIs. This approach enhances flexibility and allows teams to develop, test, and deploy services independently. An example is an e-commerce platform where product search, user profiles, and payment processing are accomplished by separate microservices.
11. How do you implement security measures in your applications?
Security measures include:
Encryption: Protects data in transit and at rest, like using TLS.
Input Validation: Ensures that user inputs do not allow injection attacks.
Continuous Monitoring: Helps detect and respond to vulnerabilities quickly.
12. Can you explain what a load balancer does?
A load balancer distributes incoming network traffic across multiple servers. This prevents any single server from becoming overwhelmed, leading to improved performance. For instance, a company experiencing a 70% increase in traffic would utilize a load balancer to ensure all servers share the load evenly.
13. What tools do you use for version control?
I primarily use Git, which allows for seamless collaboration among developers and facilitates tracking changes. Speaking of popularity, GitHub reports hosting over 100 million repositories, indicating its widespread use in the developer community.
14. How do you perform unit testing for your backend code?
I perform unit testing by writing test cases for individual functions. Tools like JUnit for Java or pytest for Python help ensure each unit of code works as expected. For example, a typical practice involves testing a login function to validate different user credentials.
15. What is Docker, and how have you used it in your projects?
Docker is a containerization tool that packages applications and their dependencies in a single container. I have utilized it to create consistent development environments. For instance, Docker allowed my team to streamline the deployment of applications across different platforms without environment discrepancies.
16. Can you explain what a database index is?
A database index speeds up data retrieval operations on a table. By creating an index on a column, queries can access rows quicker. For example, an indexed column can reduce search times from seconds to milliseconds, significantly improving application performance.
17. How do you handle errors in your backend applications?
I manage errors through structured logging and exception handling. This approach ensures that any issue is logged for debugging without disrupting the user experience. For instance, using a logging framework like Log4j allows for easy tracking of errors in Java applications.
18. What is a job queue, and why is it useful?
A job queue manages and processes tasks in the background without interrupting the user interface. This is particularly beneficial for handling tasks like sending emails or processing payments, ensuring users receive immediate feedback.
19. Discuss your experience with cloud platforms.
I have experience with cloud platforms, especially AWS and Google Cloud. For instance, I used AWS Lambda for serverless applications, allowing the code to run automatically in response to events without provisioning servers.
20. How do you ensure data integrity in your applications?
Data integrity is maintained through database constraints, using transactions to ensure atomicity, and performing regular data checks. For example, employing foreign key constraints prevents orphaned records in relational databases.
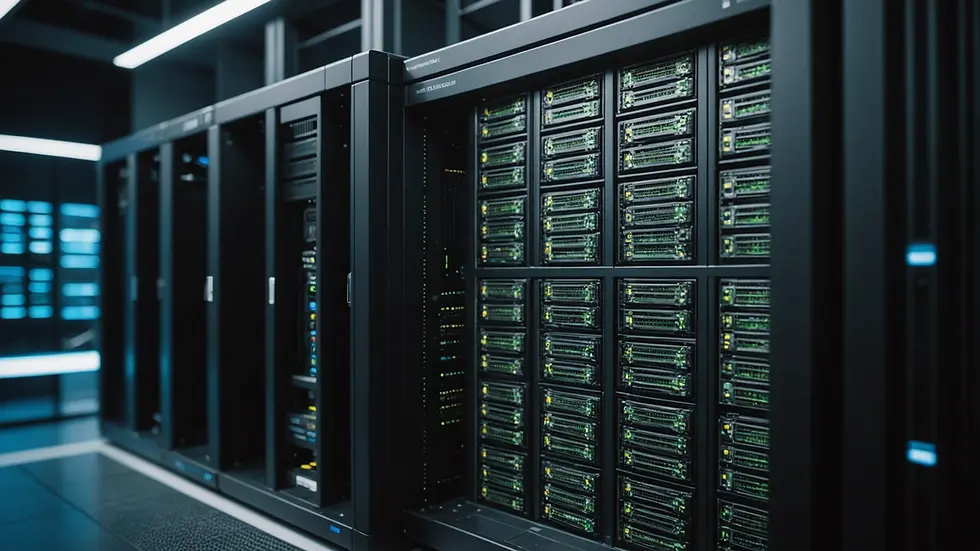
21. What are the main differences between GET and POST requests?
GET requests retrieve information and should not alter the server state, while POST requests send data to create or update resources. For instance, a form submission on a website typically uses POST to register new users.
22. How do you approach API documentation?
API documentation is crafted using tools like Swagger. These tools generate interactive documentation, allowing developers to see API endpoints and test them in real-time, significantly aiding client integration efforts.
23. What is a session, and how is it managed?
A session is a temporary interaction between a user and a server, managed by either cookies or server-side storage. For example, a login session might use a cookie to remember user credentials during their browsing session.
24. How do you design a scalable application?
Designing a scalable application involves efficient database design, load balancing, and creating microservices. This allows specific parts of the application to scale independently based on demand, accommodating a growing number of users.
25. Can you explain what a proxy server is?
A proxy server acts as an intermediary between a client and a server. It helps by hiding the client's IP address, caching content for faster access, and improving security.
26. What is the role of middleware in web development?
Middleware processes requests before they reach the server. It can handle functionalities like logging, authentication, and error management, streamlining the request-response cycle for web applications.
27. Describe the importance of logging in backend applications.
Logging is vital for monitoring application performance and diagnosing issues. Effective logging provides insights into usage patterns and helps identify areas for optimization, contributing to a better user experience.
28. How do you keep yourself updated with the latest technologies?
I stay current by following reputable tech blogs like Stack Overflow, engaging in online courses, and participating actively in coding communities. This commitment to learning helps me incorporate new technologies into my work effectively.
29. What are some common performance bottlenecks you have encountered?
Common bottlenecks include slow database queries, high memory usage, and under-resourced servers. Identifying these issues is crucial; for instance, optimizing a slow SQL query can reduce processing time by over 50%.
30. How do you handle data storage and retrieval in your applications?
I utilize appropriate database systems, optimizing retrieval with indexing and caching strategies. For example, implementing Redis for caching frequently accessed data can significantly enhance response times.
31. Can you explain the concept of a RESTful service?
A RESTful service enables stateless interactions where requests contain all necessary information for the server to process them. This design promotes scalability and simplifies server performance.
32. What is the importance of API versioning?
API versioning is crucial for introducing new features without breaking existing client applications. This practice ensures stability and backward compatibility, allowing users to access older versions as needed.
33. How do you ensure user data privacy?
User data privacy is prioritized through encryption, strict access controls, and compliance with regulations like GDPR. Implementing these measures builds user trust and protects sensitive information.
34. What is a web socket, and when would you use it?
Web sockets enable real-time communication between the client and server. They are ideally used in applications requiring instant updates, such as chat applications or live financial dashboards.
35. How do you address performance issues in your APIs?
Performance issues are tackled by optimizing database queries, compressing data payloads, and implementing caching mechanisms. By doing so, load times can improve significantly, benefiting user experience.
36. What are some challenges you face as a backend developer?
Common challenges include managing data migrations, ensuring robust security, and updating legacy systems while implementing new features. Addressing these challenges is crucial to maintaining application reliability.
37. How do you use testing frameworks in your development process?
Incorporating testing frameworks like Mocha for Node.js allows for automating unit tests and ensuring that code changes do not introduce new bugs. This practice helps maintain overall application stability and functionality.
38. What is the significance of dependency injection in backend development?
Dependency injection enables better management of dependencies, resulting in modular and easily maintainable code. This approach simplifies testing, as you can easily mock dependencies during unit testing.
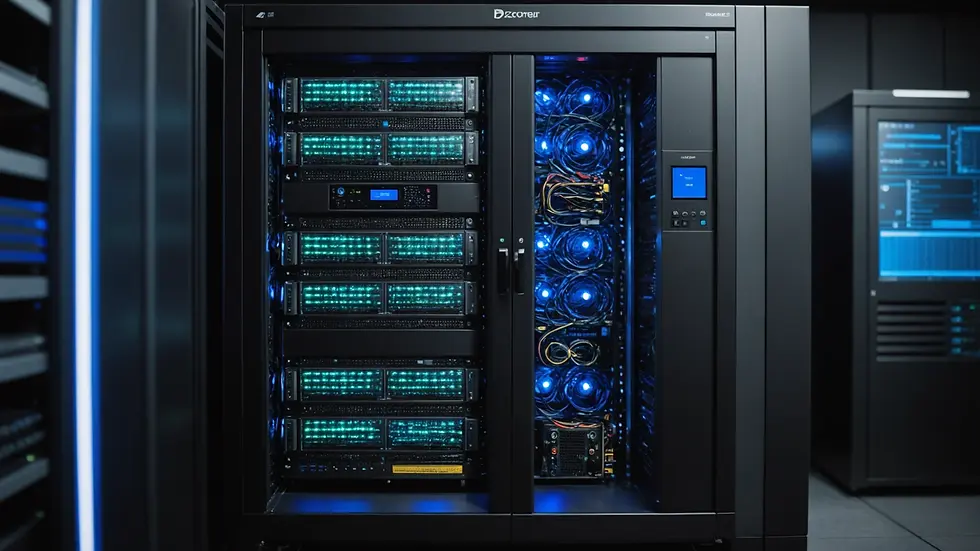
39. Can you explain what an ORM is?
An Object-Relational Mapping (ORM) tool translates data between the programming language and relational databases. For example, using an ORM like Hibernate allows developers to work with data as objects, simplifying data manipulation.
40. How do you approach debugging in your applications?
Debugging involves methodically isolating code, utilizing tools like breakpoints and log analysis to trace issues. This systematic approach often leads to quicker identification and resolution of bugs.
41. What is the role of caching in backend architecture?
Caching stores frequently accessed data to decrease load times and reduce server strain. For instance, implementing a caching layer can lead to performance improvements of up to 70%, making applications more responsive.
42. How do you handle concurrency in your backend applications?
Concurrency is managed through locking mechanisms or leveraging concurrent data structures to ensure data integrity. Using techniques like optimistic locking can help avoid conflicts in multi-user environments.
43. What is a CDN, and how does it improve performance?
A Content Delivery Network (CDN) stores content in multiple locations worldwide. By serving content from the nearest location to a user, CDNs can reduce latency and significantly enhance load times, improving user satisfaction.
44. Can you explain what a SQL injection attack is?
SQL injection attacks involve inserting malicious SQL statements into input fields to manipulate database queries. Awareness of this risk prompts developers to implement precautions like input validation and parameterized queries.
45. How do you ensure your code is maintainable?
Maintaining code involves adhering to consistent coding standards, providing comprehensive documentation, and writing modular code. For example, creating reusable components can greatly improve maintainability.
46. What is the significance of the HTTP/2 protocol?
HTTP/2 enhances website performance by allowing multiple simultaneous requests, reducing latency through multiplexing, and optimizing data transfer. This improvement can significantly speed up load times, benefiting user experience.
47. How do you handle third-party API integrations?
Third-party API integrations require careful documentation review, managing error responses, and securing proper authentication. For instance, using OAuth for social media logins ensures that users approve third-party access to their data.
48. Discuss your experience with serverless computing.
Serverless computing enables developers to run applications without managing servers. I have employed services like AWS Lambda to create event-driven applications that automatically scale based on usage, making development more efficient.
49. What are best practices for writing clean code?
Best practices for clean code include using meaningful variable names, consistent formatting, thorough comments, and following the DRY (Don't Repeat Yourself) principle to reduce redundancy.
50. How do you measure the success of your backend applications?
Success is gauged through key performance indicators (KPIs) such as:
Response times: Ideally under 200 milliseconds.
Error rates: Maintaining less than 1% error rates ensures reliability.
User satisfaction: Regular feedback can quantify user experience.
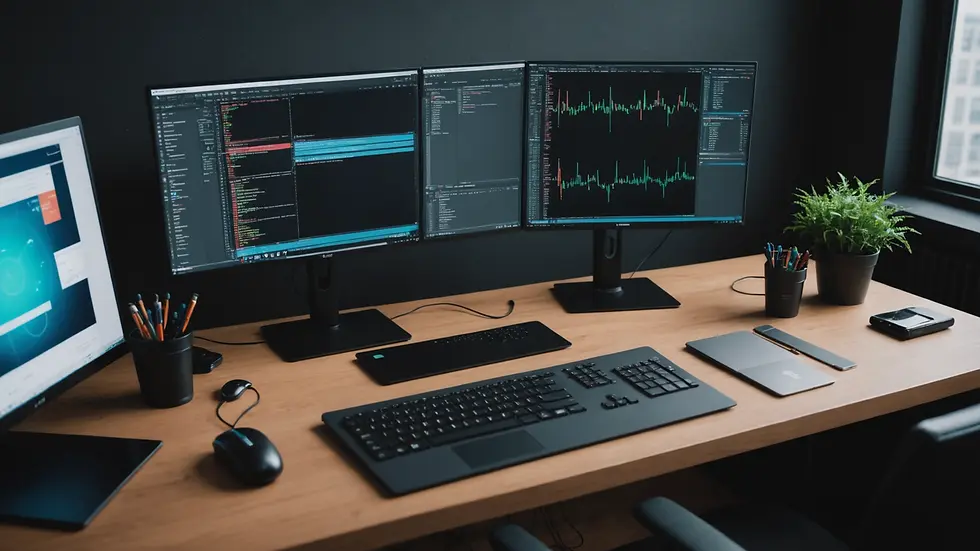
Preparation for Interview Success
As you prepare for your interview as a Backend Developer at Abridge, focus on understanding core concepts, mastering essential tools, and expressing your experiences clearly. The questions and answers provided here are meant to guide your preparation and help you approach your discussions with confidence.
Whether you're an experienced professional or new to the field, mastering these topics will significantly boost your chances of success. Stay dedicated to your learning, practice regularly, and embrace the challenges ahead. Wishing you the best of luck!