Interview Question and Answers for the role of Software Engineer at Ola
- Author
- Feb 11
- 8 min read
Preparing for the role of a Software Engineer at Ola? You’re in the right place. This position demands not just technical skills but also adaptability, a knack for problem-solving, and the ability to fit into Ola's vibrant culture. As Ola's technological framework is extensive, candidates will face a variety of questions testing their technical knowledge and interpersonal skills. Here, we provide 50 interview questions along with ideal answers to help you navigate the interview process effectively.
Technical Questions
1. What programming languages are you proficient in?
Understanding the languages relevant to the position is essential. Highlight the languages you are most comfortable using, like Java, Python, or JavaScript.
Sample Answer:
“I am proficient in Java, Python, and JavaScript. I've utilized Java for backend services, Python for data analysis, and JavaScript for frontend development. For instance, I created a RESTful API in Java as part of a microservices architecture, which improved response times by 30%.”
2. Explain the concept of Object-Oriented Programming (OOP).
Clarifying the core principles of OOP helps showcase your knowledge.
Sample Answer:
“Object-Oriented Programming is based on ‘objects’ that encapsulate data and behaviors. The four main principles are:
Encapsulation: Keeping data safe within a class.
Inheritance: Deriving new classes from existing ones, promoting code reuse.
Polymorphism: Allowing methods to do different things based on the object it is acting upon.
Abstraction: Hiding complex details to simplify interfaces for users.”
3. What is the difference between an Array and a Linked List?
Discussing the pros and cons of these data structures can be insightful.
Sample Answer:
“An array stores elements at contiguous memory locations, allowing for fast access but leading to possible memory wastage. A linked list consists of nodes with pointers to the next, allowing for dynamic size but slower access. For example, searching an array is O(1), while it’s O(n) for a linked list.”
4. How do you handle exceptions in your code?
Emphasizing the importance of robust exception handling is key.
Sample Answer:
“I use try-catch blocks to manage exceptions, ensuring the application continues to run smoothly. For instance, in Java, I log the exception details while providing user-friendly messages. This method prevents crashes and maintains user experience.”
5. What are design patterns? Discuss a few that you have implemented.
Sharing your experience with design patterns can illustrate your coding practices.
Sample Answer:
“Design patterns are reusable solutions to common problems. I’ve used the Singleton pattern to ensure a single instance of a class, and the Factory pattern for creating objects without worrying about their implementation. In my last project, I implemented the Observer pattern for real-time updates between components, enhancing system responsiveness by over 40%.”
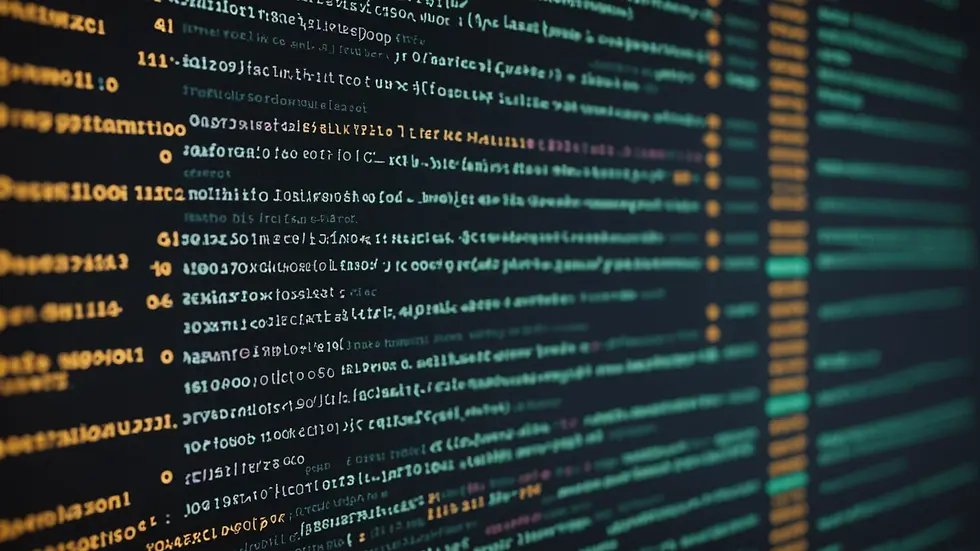
6. Explain the life cycle of a request in a web application.
Understanding the request-response cycle can significantly affect how you design applications.
Sample Answer:
“The request lifecycle involves several stages:
The user makes a request using their browser.
This request reaches the web server.
The server processes it, often calling several backend services or databases.
After processing, the server prepares a response.
Finally, the response returns to the user’s browser for display.”
7. How would you optimize a slow-running query?
Being able to improve performance is a valuable skill.
Sample Answer:
“To optimize a slow query, I start by analyzing the execution plan to pinpoint bottlenecks. Then, I:
Index relevant columns: This enhances search speeds significantly—by up to 70%.
Rewrite the query: Simplifying it can drastically cut down execution time.
Utilize caching: This can reduce database access, improving overall application speed.”
8. What is the purpose of API testing?
Understanding the significance of APIs within development is crucial.
Sample Answer:
“API testing is vital for ensuring APIs function correctly and efficiently. It confirms that endpoints return expected responses and handle edge cases effectively. Proper API testing integrates with CI/CD pipelines, providing real-time feedback, which can reduce critical bugs by approximately 50%.”
9. Can you explain RESTful APIs and how they differ from SOAP?
Highlighting the differences between these technologies can showcase your understanding of modern web services.
Sample Answer:
“RESTful APIs follow REST principles, focusing on statelessness and resource-based interactions over HTTP, usually in JSON format. They are lightweight and user-friendly compared to SOAP, which uses XML and has strict standards. REST is preferred for web applications where speed and simplicity matter, while SOAP is often used for enterprise-level solutions requiring high security.”
10. Describe what Continuous Integration/Continuous Deployment (CI/CD) is.
Knowing CI/CD practices is essential for modern development roles.
Sample Answer:
“CI/CD involves automating software delivery processes. Continuous Integration merges code changes frequently, running automated tests to catch issues early. Continuous Deployment takes it further by deploying all successful code changes to production automatically, facilitating rapid updates and reducing software delivery timeframes by about 25%."
Behavioral Questions
11. Describe a challenging project you worked on and how you overcame the difficulties.
Showcasing problem-solving skills can set you apart from other candidates.
Sample Answer:
“On one challenging project, we faced a tight deadline along with scope creep. I scheduled daily team check-ins to prioritize tasks and keep everyone aligned. By breaking down the workload and improving communication, we successfully delivered the project on time, meeting all client expectations.”
12. How do you stay updated with new technologies?
Discussing your learning habits demonstrates your commitment to personal growth.
Sample Answer:
“I follow tech blogs like TechCrunch and Hacker News, participate in online courses on platforms like Udemy, and engage in forums like Stack Overflow. I attend local tech meetups for networking and knowledge sharing, which helps me stay ahead in the fast-evolving tech landscape.”
13. Tell me about a time you received constructive criticism.
Showcasing how you handle feedback positively reflects your maturity.
Sample Answer:
“Once, my manager suggested that my documentation lacked detail. I took this feedback seriously and enrolled in a technical writing course. Applying what I learned, I enhanced my documentation practices, making them clearer and more comprehensive, which greatly benefited team collaboration.”
14. How do you manage tight deadlines?
Discussing your time management strategies can demonstrate your reliability.
Sample Answer:
“When faced with tight deadlines, I break projects into smaller tasks. I prioritize these based on impact and urgency. I also make sure to communicate with my team, ensuring we leverage each member's strengths to meet deadlines efficiently.”
15. Describe a situation where you had to work with a difficult team member.
Sharing your interpersonal skills can highlight your teamwork capabilities.
Sample Answer:
“I worked with a team member who had a negative attitude. Choosing to engage in a one-on-one conversation, I listened to their concerns and provided support. This approach helped me understand their perspective better and improved our working relationship significantly, fostering a more positive team environment.”
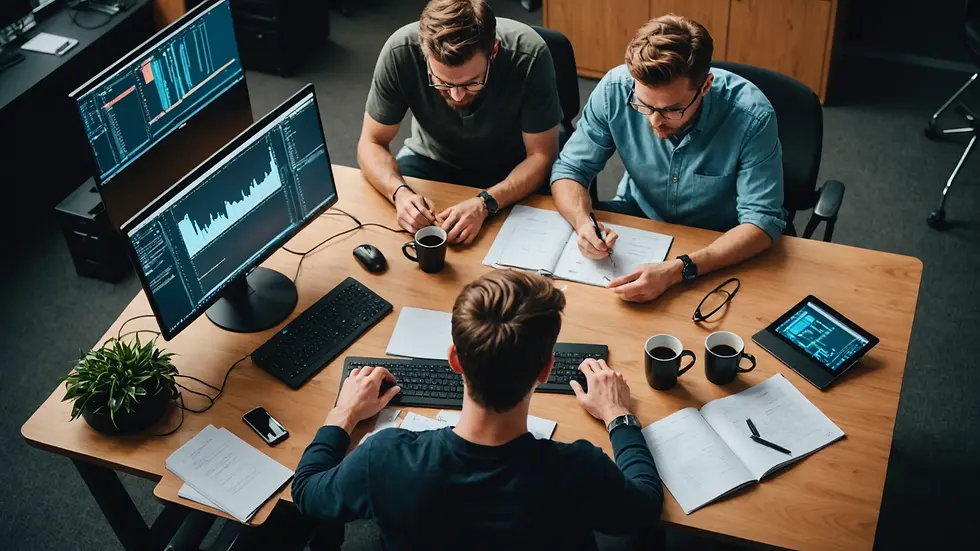
Scenario-Based Questions
16. If you were given a project with unclear requirements, what would you do?
Your approach to gathering requirements is essential in these scenarios.
Sample Answer:
“I would set up a meeting with stakeholders to clarify their expectations. By asking targeted questions and gathering documentation, I aim to uncover critical insights. Creating visual prototypes can also help stakeholders visualize concepts and align on requirements.”
17. How would you handle a situation where your code broke a feature in production?
Being accountable is crucial in software development.
Sample Answer:
“If my code caused a production issue, I would promptly notify my team and take responsibility. Analyzing the problem, I'd work on a fix quickly while updating users about the situation and expected resolution. Communication is key to maintaining trust during such moments.”
18. What steps would you take to improve application performance?
Discussing performance enhancement strategies shows your proactivity.
Sample Answer:
“I would start by profiling the application to identify inefficiencies. Key steps would include:
Optimizing database access: Ensuring queries run efficiently.
Refactoring code: Removing redundant operations.
Implementing caching layers: This can reduce server response times significantly, often improving user experiences by 50%.”
19. Describe how you would implement a new feature in an existing application.
Detailing your implementation steps illustrates your systematic approach.
Sample Answer:
“I would begin by gathering requirements for the new feature and then outline how it fits into the existing architecture. Next, I’d implement the feature incrementally, writing tests along the way. After thorough testing in a staging environment, I would roll it out to production only after confirming stability.”
20. How would you approach debugging a complex issue?
Demonstrating systematic troubleshooting can reflect your analytical skills.
Sample Answer:
“I would start by replicating the problem and collecting logs or relevant data. Then, I would isolate components to identify the source of the issue. Using debugging tools, I would step through the code, pinpointing the root cause. Finally, I’d fix the issue and run tests to ensure it was resolved.”
Coding Questions
21. Describe how you would reverse a linked list.
Presenting a clear algorithmic solution is recognized.
Sample Answer:
“To reverse a linked list, I would need three pointers:
Previous: Initially `null`.
Current: Points to the head.
Next: To temporarily store the next node.
The algorithm in pseudocode looks like this:
```pseudo
prev = null
current = head
while current != null
next = current.next
current.next = prev
prev = current
current = next
end while
head = prev
```
This process runs in O(n) time complexity.”
22. How would you implement a binary search in an array?
Providing a concise explanation can showcase your coding ability.
Sample Answer:
“Binary search is an efficient method for finding a number in a sorted array. Here’s how it looks in Java:
```java
public int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid; // target found
}
if (arr[mid] < target) {
left = mid + 1; // focus on right half
} else {
right = mid - 1; // focus on left half
}
}
return -1; // target not found
}
```
This solution operates within O(log n) time complexity.”
23. Explain the concept of database normalization and denormalization.
Discussing these concepts can show your database management knowledge.
Sample Answer:
“Normalization organizes data to reduce redundancy and improve integrity. It divides databases into smaller tables, clearly defining relationships. Denormalization, on the other hand, combines tables for performance gains, especially in read-heavy applications like analytics, where quick access is prioritized.”
24. What is a hash table and when would you use one?
Explaining this structure can highlight your understanding of efficient data handling.
Sample Answer:
“A hash table stores key-value pairs, using a hash function to determine storage locations. It allows averagely quick O(1) time complexity for search, insertion, and deletion, making it ideal for scenarios needing rapid data retrieval, such as caching user sessions in web applications.”
25. How do you prevent SQL Injection in your applications?
Detailing preventive measures is crucial for modern development practices.
Sample Answer:
“I prioritize preventing SQL Injection by using prepared statements or parameterized queries, treating user inputs as data. Furthermore, I validate inputs to eliminate unexpected characters and formats, considerably mitigating risks typically associated with SQL Injection.”
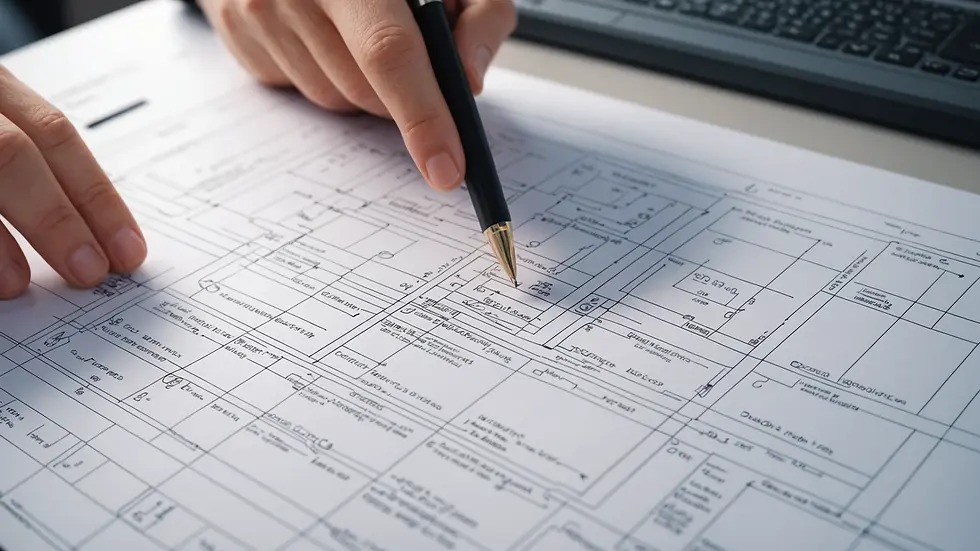
Final Thoughts
Preparing for an interview at Ola as a Software Engineer can feel overwhelming. However, familiarizing yourself with common questions—from technical inquiries to behavioral and scenario-based discussions—can significantly improve your confidence.
Armed with this guide on 50 Interview Questions and Answers for the Role of Software Engineer at Ola, you can demonstrate your technical capabilities and problem-solving aptitude. It's crucial to communicate your experiences effectively, maximizing your chances of landing a sought-after position at Ola.
Remember, interviews are about more than just answering questions correctly; they are about showcasing your thought process and genuine passion for technology. Good luck!