Interview Questions and Answers for Software Development at Adobe
- Author
- Feb 11
- 8 min read
When preparing for a software development interview at Adobe, it's essential to anticipate the types of questions you may encounter. This comprehensive guide provides 50 interview questions and answers specifically tailored for prospective software developers looking to land a position at one of the world's leading technology companies.
This guide aims to equip you with the tools needed to showcase your technical skills and your understanding of software development basics, project management, teamwork, and problem-solving abilities.
Understanding Adobe’s Interview Process
Adobe's interview process typically involves multiple stages, including an initial screening, technical interviews, and behavioral interviews. During this process, candidates may be assessed on their coding skills, problem-solving abilities, and cultural fit.
Anticipating the types of questions you may face can significantly boost your confidence and readiness for the interview day.
Technical Questions
1. What is the difference between a process and a thread?
A process is an independent program that runs in its own memory space, while a thread is a smaller sequence of instructions that can be managed independently and shares the memory space of the process.
2. What are the main principles of Object-Oriented Programming (OOP)?
The main principles of OOP are encapsulation, inheritance, polymorphism, and abstraction, which enable developers to create modular, reusable code.
3. Can you explain the concept of a linked list?
A linked list is a linear data structure where each element (node) contains a data field and a reference (or link) to the next node in the sequence, allowing for efficient insertion and removal.
4. How would you manage version control in a collaborative environment?
Implementing a version control system like Git allows multiple developers to work on different branches, track changes, and easily merge code without conflicts.
5. What is the significance of unit testing?
Unit testing helps in validating individual components of code to ensure they function as expected, leading to early detection of bugs and more maintainable code.
6. Describe a situation where you had to troubleshoot a complex issue.
When faced with a complex issue, I would first gather information about the problem, then attempt to isolate it by checking logs and histories, followed by reproducing the bug, and finally applying a methodical approach to test potential solutions.
7. Explain the concept of API and its role in software development.
An API (Application Programming Interface) is a set of rules that allows different software entities to communicate, serving as the bridge for different components to interact, thereby enhancing modularity and scaling.
8. What is Big O notation, and why is it important?
Big O notation is a mathematical notation that describes the performance or complexity of an algorithm in relation to the input size, helping developers in evaluating how an algorithm scales.
9. Can you differentiate between SQL and NoSQL databases?
SQL databases are relational and use structured query language for querying, while NoSQL databases are non-relational and store data in a flexible format, often making them suitable for large-scale data types.
10. Describe a time you had to work with a legacy codebase.
While working with a legacy codebase, I focused on understanding the existing architecture, isolated sections for gradual refactoring, and ensured adequate documentation to facilitate updates.
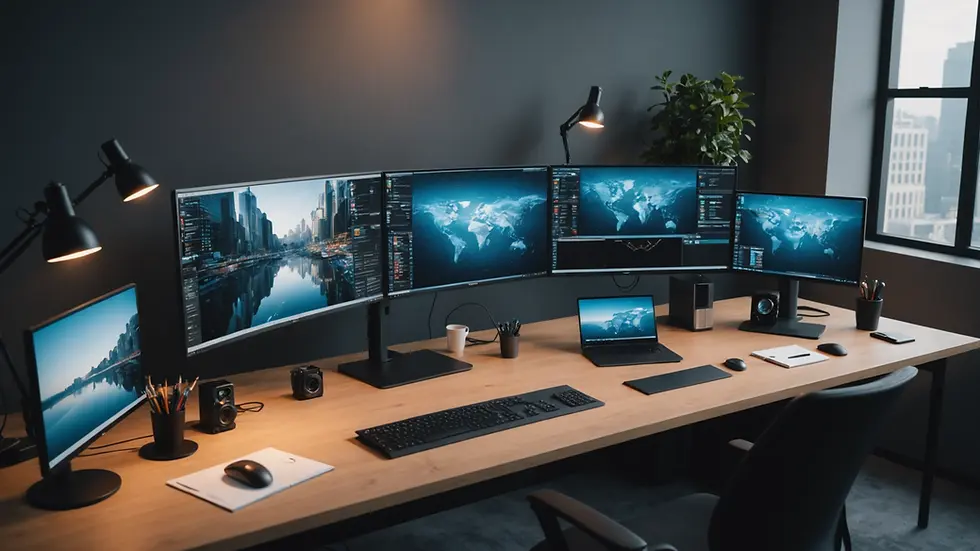
Behavioral Questions
11. How do you prioritize tasks in a project?
I prioritize tasks based on their urgency and impact on project timelines, often using techniques like the Eisenhower matrix to categorize tasks effectively.
12. Can you give an example of how you handled a conflict within a team?
When faced with conflict, I initiated a discussion to address concerns openly, focused on common goals, and facilitated a collaborative approach to find a mutually beneficial solution.
13. How do you handle tight deadlines?
I handle tight deadlines by breaking down projects into manageable tasks, estimating time requirements accurately, and maintaining clear communication with my team and stakeholders.
14. What motivates you to work in software development?
The continuous challenge of solving complex problems, the rapid evolution of technology, and the opportunity to build products that can positively impact users motivate me continuously.
15. Describe your experience with Agile methodologies.
I have worked extensively with Agile methodologies, participating in daily stand-up meetings and sprint planning, which has helped foster collaboration and adaptability within my teams.
16. How do you ensure quality in your code?
I ensure quality through practices such as regular code reviews, writing unit tests, adhering to coding standards, and utilizing automated testing tools.
17. Describe a project that you are particularly proud of.
I developed a mobile application that streamlined internal communications, leading to a 30% increase in team collaboration, which was both a challenging and rewarding experience.
18. How do you stay updated with the latest software development trends?
I keep updated by attending conferences, participating in online forums, reading industry blogs, and following key thought leaders on digital platforms.
19. What is your approach to mentoring others in your team?
My approach involves offering constructive feedback, sharing resources, and encouraging open dialogue to help team members grow in their technical skills and confidence.
20. How do you deal with feedback?
I see feedback as a learning opportunity. I carefully assess it, reflect on its value, and take actionable steps to improve my work based on the insights gained.
System Design Questions
21. How would you design a URL shortening service?
I would use a database to store original URLs and their shortened counterparts, implement hashing algorithms for generating short links, and create an API for users to retrieve links.
22. Explain the working of a load balancer.
A load balancer distributes incoming traffic among multiple servers to ensure no single server is overwhelmed, improving availability and reliability.
23. How do you ensure fault tolerance in your applications?
I ensure fault tolerance by implementing redundancy, failover mechanisms, and monitoring systems to detect issues early and maintain service continuity.
24. Describe how you would implement pagination in a web application.
I would implement pagination by determining the current page, fetching the total count of records, and limiting the number of records per request, presenting them efficiently to the user.
25. What factors would you consider while designing a scalable system?
Factors include load balancing, database performance, caching mechanisms, network latency, and redundancy to handle varying loads effectively.
26. How would you handle data consistency in a distributed system?
I would apply techniques such as eventual consistency, distributed transactions, and consensus protocols like Paxos or Raft to ensure data integrity across nodes.
27. Explain the importance of caching in web applications.
Caching is crucial for improving application performance and scalability by temporarily storing frequently accessed data, reducing load times and database queries.
28. How do you manage state in a web application?
State can be managed through sessions, cookies, or client-side storage solutions (like localStorage) to preserve user data as they navigate across pages.
29. What is microservices architecture?
Microservices architecture is an approach where applications are structured as a collection of loosely coupled services, each responsible for specific functionalities, enhancing scalability and flexibility.
30. Describe how you would approach optimizing a slow application.
I would analyze performance metrics, investigate bottlenecks, consider optimizing database queries, implementing caching strategies, and reviewing application architecture for inefficiencies.
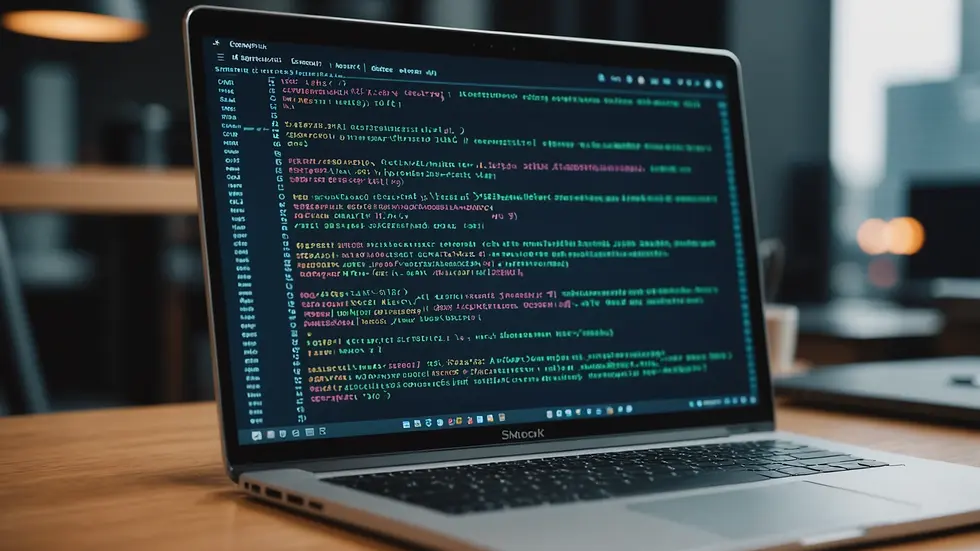
Follow-Up Questions
31. What are your thoughts on pair programming?
Pair programming fosters collaboration, allows for real-time code review, and can lead to higher-quality code as two developers share different perspectives and expertise.
32. How do you approach technical debt?
I prioritize addressing technical debt by allocating time in sprints for refactoring, reviewing legacy code, and ensuring that new implementations adhere to coding standards to prevent future debt.
33. Can you explain a time you introduced a new technology in a project?
I introduced a CI/CD pipeline in a project, which streamlined our deployment process, reduced integration issues, and enhanced our overall development speed and reliability.
34. What coding languages are you most comfortable with, and why?
I am most comfortable with Python and JavaScript due to their versatility and strong community support, which makes developing a range of applications more efficient.
35. Describe your approach to data security.
My approach includes implementing encryption, conducting regular security audits, adhering to best practices, and educating team members on secure coding techniques.
36. How would you improve collaboration among remote team members?
I would implement effective communication platforms, establish regular check-ins, and promote a culture of transparency to ensure all members are aligned and engaged regardless of location.
37. What steps do you take when estimating project timelines?
I break down tasks into detailed sub-tasks, consult with team members for input, analyze previous project durations, and build in buffers for unforeseen issues.
38. How do you handle stress and burnout in the workplace?
I prioritize work-life balance, practice mindfulness, and engage in hobbies or physical activities to recharge and maintain a positive attitude.
39. Can you describe your approach to learning a new programming language?
I begin by understanding the fundamentals and syntax, then progress through hands-on projects and online resources, and engage with communities for further learning and support.
40. How do you deal with ambiguity in requirements?
I seek clarification from stakeholders to gather more details and context, and if necessary, I prioritize feedback loops to adapt to changing requirements as they become clearer.
Culture Fit Questions
41. Why do you want to work for Adobe?
I admire Adobe’s commitment to creativity and innovation and want to contribute to projects that empower users to express themselves through technology.
42. Describe an environment where you thrive the most.
I thrive in an environment that encourages innovation, collaboration, and open communication, where team members feel empowered to share ideas and take initiatives.
43. How do you handle differing opinions within the team?
I promote respectful discussions where all viewpoints are considered. If necessary, I facilitate a decision-making process that draws on the team's collective expertise.
44. What role do you usually take on in group settings?
I often take on the role of a mediator, ensuring all voices are heard and facilitating collaboration towards our common goals.
45. How do you contribute to a positive team atmosphere?
I contribute by fostering open communication, encouraging team bonding activities, and recognizing teammates’ achievements, which helps build morale and a strong team dynamic.
46. Can you describe a time when you were a part of a diverse team?
In a previous project, my team comprised members from various backgrounds. We successfully leveraged individual strengths, leading to creative solutions and a rich exchange of ideas.
47. How do you align your work with company values?
I familiarize myself with the company's values and mission, ensuring my projects reflect those principles and contribute to the company's overall goals.
48. What does a successful team mean to you?
A successful team is one that communicates openly, collaborates effectively, supports each other’s growth, and collectively achieves its objectives while maintaining a positive environment.
49. Describe a situation where you had to learn from failure.
In a project that did not meet expectations, I took time to analyze what went wrong, embraced the lessons learned, and implemented changes in our approach for future success.
50. What are your career aspirations in software development?
I aspire to continue learning and growing in my technical skills, take on leadership roles, and contribute to building innovative solutions that still have a significant impact.
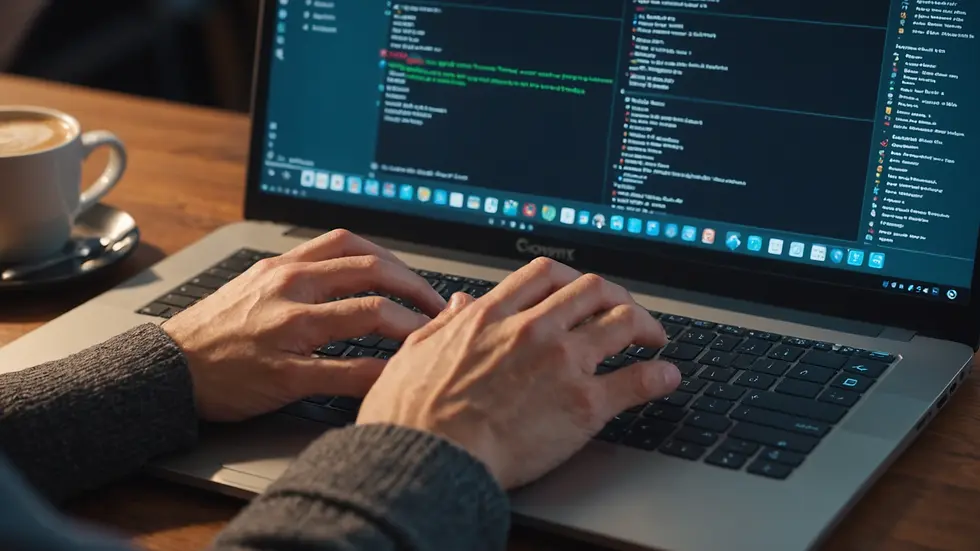
Conclusion
Preparing for an interview at Adobe for a software development role requires a solid understanding of both technical and behavioral aspects of the job. This guide has provided you with 50 interview questions and answers that will help you build a strong foundation for a successful interview.
Incorporating insights on technical principles, teamwork, and problem-solving abilities will help you effectively demonstrate your skills and readiness for the challenges ahead. Best of luck as you embark on your journey to joining the innovative team at Adobe!