Interview Questions and Answers for Software Development at Google
- Author
- Feb 11
- 6 min read
Preparing for an interview with a tech giant like Google is an exhilarating journey. With a hiring process that is notoriously tough, candidates must be ready for challenging technical questions and should demonstrate strong problem-solving skills. This post provides a comprehensive list of 50 interview questions along with answers, specifically curated for software development roles at Google. From core concepts in data structures and algorithms to system design and behavioral queries, this guide will equip you with essential knowledge and tips for success.
1. General Technical Questions
What is a data structure?
A data structure is a method of organizing and storing data so that it can be accessed and modified efficiently. Common examples are arrays, linked lists, stacks, queues, trees, and graphs. For instance, a binary search tree allows for faster searches compared to a plain list.
What are the differences between an array and a linked list?
Arrays have a fixed size and allow indexed access, making random access simple and fast. In contrast, linked lists are dynamic structures where each node points to the next one, allowing for efficient insertions and deletions. For example, inserting an element in the middle of a linked list is O(1), whereas in an array it requires shifting elements, making it O(n).
Explain the concept of recursion. Give an example.
Recursion involves a function calling itself to solve smaller instances of a problem. A classic example is calculating the factorial of a number, where `factorial(n) = n * factorial(n-1)` with a base case of `factorial(0) = 1`. This approach is elegant for problems defined in terms of smaller subproblems.
What are the advantages of using a hash table?
Hash tables offer average-case time complexities of O(1) for insertions, deletions, and lookups, making them very efficient. However, effectiveness depends on a well-designed hash function to reduce collisions. For example, if you need to store hundreds of thousands of users and quickly access them, a hash table is ideal.
Can you explain Big O notation?
Big O notation describes how the performance of an algorithm changes as the input size grows, giving insight into its efficiency. For example, an algorithm that runs with time complexity O(n^2) will scale poorly with large inputs, whereas O(log n) algorithms remain efficient even as data size increases.
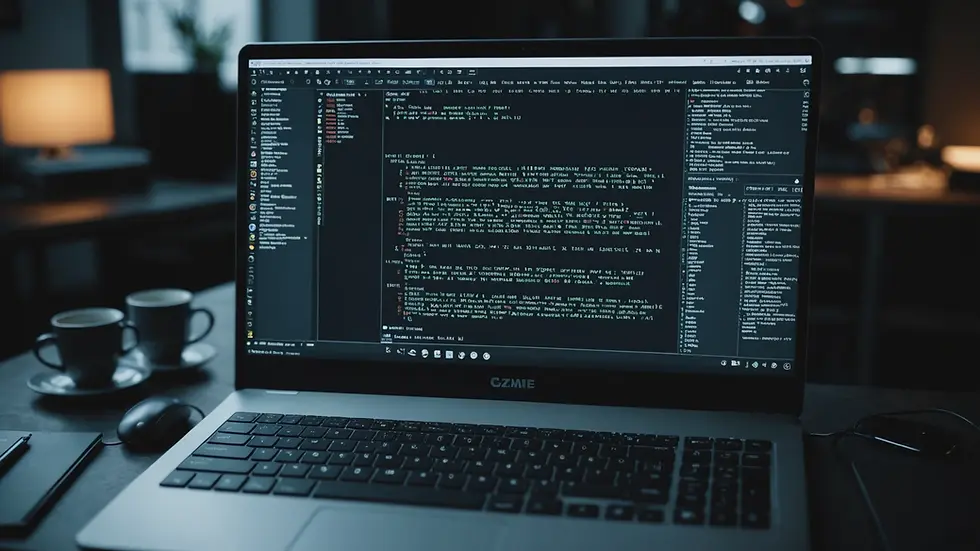
2. Algorithms and Problem Solving
How would you reverse a linked list?
To reverse a linked list, initialize three pointers: `prev`, `current`, and `next`. As you traverse the list, redirect each `next` pointer to point to `prev`, effectively reversing the list's direction. This modification runs in O(n) time.
What is dynamic programming?
Dynamic programming is a powerful technique for solving problems by breaking them down into simpler overlapping subproblems and storing their results. A famous example is the Fibonacci sequence, where the nth Fibonacci number can be computed efficiently by using previously computed values.
Can you provide an algorithm for finding the largest element in an array?
You can find the largest element by iterating through the array with a single loop, keeping track of the maximum value encountered. This algorithm runs in O(n) time. For example, in an array of 100 elements, you only need to check each one once.
What are the characteristics of a binary search tree (BST)?
A BST allows each node to have up to two children, with the left child containing values less than the parent and the right child containing values greater. This property enables efficient operations like search, insert, and delete with average O(log n) time complexity if balanced.
10. Describe a situation where a greedy algorithm might not work.
Greedy algorithms may yield suboptimal results in certain scenarios. For instance, in the coin change problem, selecting the largest denomination first does not always lead to the minimum number of coins. Instead, using dynamic programming can provide an optimal solution.
3. System Design Questions
11. How would you design a URL shortening service like bit.ly?
To create a URL shortening service, you can use a relational database to store the original URLs and generate unique keys (or slugs) for each URL. Using techniques like Base62 encoding for short keys allows for efficient storage and retrieval. For instance, a database could handle millions of entries with quick lookups.
12. What are the key considerations when designing a caching system?
Important considerations include choosing what data to cache, selecting a caching strategy (e.g., Least Recently Used), and implementing cache eviction policies to maintain performance. Additionally, ensuring consistency between cached and source data is crucial to prevent stale results.
13. Describe how you would implement a messaging queue.
A messaging queue can be implemented using a data structure to hold messages alongside methods for producing and consuming messages. Features such as ensuring message delivery, handling concurrency, and persistence become critical in a production setting. For example, systems like RabbitMQ or Kafka implement these principles effectively.
14. Explain how load balancing works.
Load balancing evenly distributes incoming network traffic across multiple servers to prevent any single server from being overwhelmed. This can improve application uptime and reliability. Techniques like round-robin and least connections are commonly used to manage traffic efficiently.
15. What is microservices architecture?
Microservices architecture structures an application as a collection of small independent services that communicate through well-defined APIs. This approach enhances scalability; for example, if one service experiences high traffic, it can scale independently without affecting the entire system.
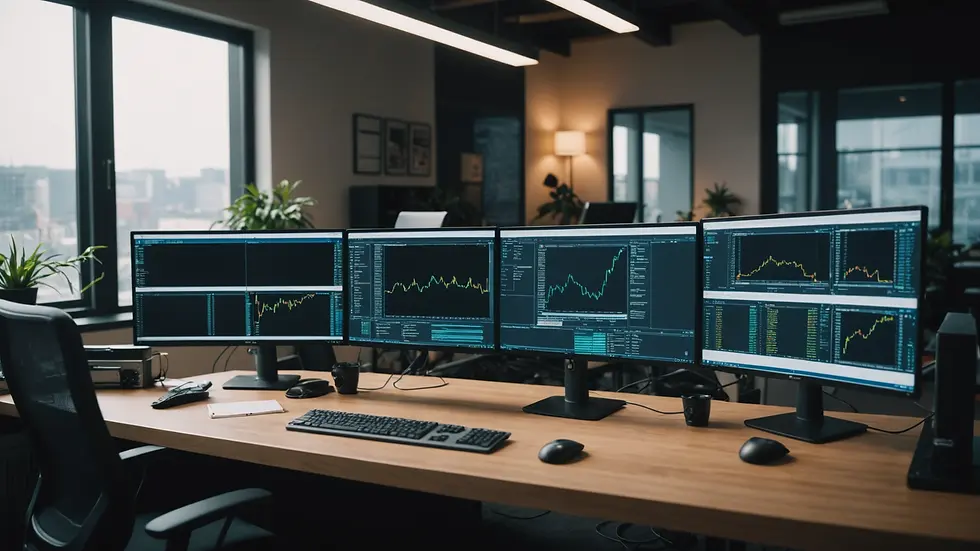
4. Behavioral Questions
16. Can you tell me about a time when you faced a significant challenge at work?
Candidates should share a specific challenge, detailing the situation, the actions taken, and the outcome. For example, discussing a tight project timeline and how collaboration and creative problem-solving led to on-time delivery can demonstrate resilience.
17. Describe a project where you took the lead. What was your approach?
Highlight an experience that showcases leadership, teamwork, and strategic planning. Mention specific roles and outcomes that exhibit your ability to drive successful results, such as improving efficiency by a certain percentage through team initiatives.
18. How do you prioritize your tasks when working on multiple projects?
Explain your prioritization strategies, using methods such as assigning deadlines and assessing project goals. For instance, you might use the Eisenhower matrix to distinguish between urgent and important tasks, enabling effective time management.
19. Have you ever disagreed with a teammate? How did you handle it?
Discuss a real experience resolving conflict positively. Describe how you actively listened to the teammate's perspective, reached a compromise, or facilitated a discussion that led to a constructive outcome.
20. What motivates you in your work?
Share intrinsic motivators, such as a passion for problem-solving or the desire to positively impact user experiences. This can accentuate your commitment to the role and align with the company's mission.
5. Language-Specific Questions
21. What are the main features of object-oriented programming?
Key features include encapsulation (bundling data and methods), inheritance (defining new classes based on existing ones), polymorphism (methods behaving differently based on the object), and abstraction (simplifying complex realities). These features enhance code organization.
22. What is a promise in JavaScript?
A promise represents a value that may be available now, later, or never for an asynchronous operation. Promises allow developers to chain operations using methods like `.then()` and `.catch()`, facilitating cleaner code and easier error handling.
23. How do you manage memory in Python?
Python uses automatic garbage collection to free unused memory. Developers can monitor memory usage and optimize data structures using profiling tools like `memory_profiler`. For high-load applications, utilizing efficient data types is essential to minimize memory overhead.
24. Explain the concept of closures in JavaScript.
A closure is a feature where a function retains access to its lexical scope, even when executed outside that scope. This is useful for state management, such as creating private variables in an object.
25. What is the difference between synchronous and asynchronous programming?
Synchronous programming runs tasks in order, blocking further tasks until completion. In contrast, asynchronous programming allows multiple tasks to run concurrently, greatly improving efficiency in I/O bound scenarios.
6. Best Practices and Coding Questions
26. What is your coding process when starting a new problem?
A sound coding process involves understanding problem requirements, brainstorming solution strategies, writing pseudocode, and iterating to improve the chosen solution. This structured approach ensures clarity and efficiency.
27. How do you ensure your code is maintainable?
Ensure maintainability through clear naming conventions, consistent commenting, adherence to coding standards, and implementing unit tests to verify functionality. For instance, writing tests increases confidence during code changes and reduces bugs.
28. What is code refactoring and why is it important?
Code refactoring involves restructuring existing code without altering its external behavior. It boosts clarity, reduces complexity, and fosters easier maintenance. For example, regularly refactoring a codebase can maintain a clean architecture over time.
29. Explain the significance of version control.
Version control systems, such as Git, track code changes over time, facilitating collaboration among multiple developers. They allow for tracking project history and easy reversion to earlier versions, which is crucial in team settings.
30. How do you handle situations where you don’t know the answer during an interview?
Acknowledge the question, and explain how you would approach finding the solution. This demonstrates humility and a willingness to learn, which are valuable qualities in any candidate.
Wrapping It Up
Preparing for a software development interview at Google requires a solid understanding of both technical concepts and interpersonal skills. The questions and answers presented here serve as a robust guide to familiarize you with potential topics while ensuring you engage clearly and confidently during discussions. By effectively showcasing both your technical expertise and personal strengths, you can significantly improve your chances of success in this competitive environment.
Use these insights to enhance your preparation for your upcoming interview at Google or any other leading tech company.