Interview Questions and Answers for Software Development at Microsoft
- Author
- Feb 11
- 8 min read
Securing a software developer position at Microsoft is an exciting challenge. The competition is fierce, and knowing what to expect can make a significant difference in your preparation. This guide lays out 50 common interview questions with tailored answers crafted for software development roles at Microsoft. By practicing these questions, you can approach your interview with more confidence and clarity.
In addition to the questions, you'll gain insight into the skills and attributes Microsoft values in its software developers.
Understanding the Interview Process
Microsoft’s interview process usually consists of multiple stages. You can expect technical interviews, behavioral assessments, and sometimes coding challenges. According to various candidate reports, about 70% of applicants encounter both technical and behavioral questions. These assess not only coding abilities but also problem-solving skills and knowledge of software engineering principles.
Microsoft also evaluates cultural fit and teamwork proficiency. Be ready to showcase both your technical expertise and your ability to collaborate and innovate.
Common Interview Questions and Answers
Here are 50 common interview questions categorized for easy reference.
Technical Skills Questions
What programming languages are you most comfortable with?
Answer: "I am proficient in languages such as C#, Java, and Python. For example, I developed a web application using C# and ASP.NET that handled over 10,000 user requests daily."
Can you explain the differences between object-oriented programming (OOP) and functional programming?
Answer: "OOP emphasizes objects that encapsulate data and behaviors, promoting reusability. For instance, I used OOP principles in a Java project to build a banking application, allowing for different account types to utilize shared methods. On the other hand, functional programming focuses on function use and immutability. I've used Python’s functional programming features, such as map and filter, to process data streams effectively."
What is your understanding of design patterns, and can you name a few?
Answer: "Design patterns are reusable solutions to common software design problems. Examples include the Singleton pattern for managing a single instance of a class, the Observer pattern for event handling, and the Factory pattern for creating objects. Using the Strategy pattern in an e-commerce system helped modularize different payment methods."
How do you conduct debugging of your code?
Answer: "I approach debugging by first reproducing the issue. For example, I once faced a bug in a production system, so I utilized logging to identify the function causing the error. This systematic approach allows me to isolate and resolve problems effectively."
What are some common algorithms you have implemented, and what are their use cases?
Answer: "I have implemented algorithms like quicksort for efficient data sorting, which I applied while optimizing database queries that saw a 50% reduction in execution time. Additionally, I used Dijkstra's algorithm for routing in a mapping application."
Behavioral Questions
Describe a challenging project you worked on and how you overcame obstacles.
Answer: "I worked on integrating a third-party payment API into our application. We faced technical documentation issues, but by setting up weekly meetings with the provider and creating a detailed integration guide, we launched the feature on time."
How do you handle conflicts with team members?
Answer: "I advocate for open discussions. For instance, when a group member and I had differing views on coding practices, we held a meeting to share our perspectives. This led to a compromise that improved our coding standards."
What motivates you as a software developer?
Answer: "I thrive on solving complex problems. In my previous role, I developed a feature that increased user engagement by 20%. This direct impact on real users motivates me to keep learning and improving."
Can you give an example of when you had to learn a new technology quickly?
Answer: "During a project transition to Azure, I dedicated 15 hours over a week to online courses and practical exercises. This rapid learning helped me to lead the migration team successfully, meeting our deadline."
10. How do you prioritize tasks when working on multiple projects?
Answer: "I list tasks based on deadlines and impact. I utilize the Eisenhower Matrix to determine critical versus non-urgent tasks. This approach helped me juggle two concurrent projects effectively last quarter."
Coding Challenges
11. Given an array of integers, find two numbers such that they add up to a specific target. How would you solve this?
Answer: "I would use a hash map to store integers as I iterate through the array. If the complement (target - current integer) exists in the map, I can achieve this with O(n) time complexity."
12. How do you reverse a linked list?
Answer: "I use three pointers: previous, current, and next. As I traverse the list, I redirect the current node’s next pointer to the previous node, thus reversing the list iteratively."
13. Can you explain how to implement a binary search algorithm?
Answer: "In a sorted array, I start with two pointers at the ends. By calculating the mid-point and comparing it to the target, I adjust the pointers until the target is found or they cross, making this search O(log n)."
14. What is your approach to optimize a SQL query?
Answer: "I first analyze the execution plan to identify bottlenecks. After adding necessary indexes, I filter early in the query process. For example, optimizing a query reduced its runtime from 8 seconds to under 2 seconds."
15. Describe how you would implement a simple web server.
Answer: "Using Express in Node.js, I would set up routes for varied HTTP methods and responses, ensuring that static files are served efficiently and client requests handled effectively."
System Design Questions
16. How would you design a URL shortening service like Bitly?
Answer: "I would create a database table to map long URLs to short ones using a hashing function. The service would also include analytics features to track user clicks and activities."
17. Describe the design considerations for a real-time chat application.
Answer: "Considerations would include using WebSockets for instant message delivery, implementing user status indicators, and maintaining secure data transfer. This design would ensure reliable user interaction."
18. What steps would you take to ensure a web application is secure?
Answer: "I would implement HTTPS for secure communication, validate input to avoid SQL injection, and conduct regular security audits. Using OAuth for authentication increases data safety further."
19. How would you architect an image processing service?
Answer: "Adopting microservices, I would separate upload handling, processing, and storage services. Using a queue for processing could help manage workloads during peak times."
20. What strategies would you use to handle large volumes of data?
Answer: "I would implement data sharding for DB efficiency, use caching for recurrent queries, and design ETL processes to optimize data storage, ensuring that access is quick and scalable."
Situational Questions
21. What would you do if you received negative feedback from a code review?
Answer: "I would appreciate the feedback, then analyze it for insight. Clarifying misunderstandings with my reviewer would help me grow and improve my coding practices moving forward."
22. How would you respond if a project you are leading underperforms?
Answer: "I would analyze the reasons for underperformance, gathering team feedback. I would then restructure our approach, focusing on clear goals to steer the project back on track."
23. Imagine you’re tasked with a project that is outside your expertise. What steps would you take?
Answer: "I would conduct thorough research on the technology, reach out to experienced colleagues for advice, and engage in hands-on learning through small projects to build confidence."
24. Describe how you would handle meeting a tight deadline.
Answer: "I would break the project into manageable tasks, assign responsibilities, and hold daily stand-up meetings for progress updates. This method keeps the team aligned and productive."
25. What would you do if you discovered a critical bug just before release?
Answer: "I would evaluate the bug’s severity and communicate with the team. Depending on the impact, I would either find an immediate fix or suggest delaying the release to ensure quality."
Questions About Processes and Tools
26. What development tools do you prefer, and why?
Answer: "I primarily use Visual Studio for C# development due to its robust features. Git is my go-to for version control, as it facilitates collaboration and efficient tracking of changes."
27. How do you ensure code quality in your projects?
Answer: "I prioritize writing unit tests and conducting regular code reviews to maintain standards. Automating testing pipelines has proven invaluable in enhancing our code quality metrics."
28. What is the importance of Agile methodology in development?
Answer: "Agile allows teams to adapt quickly to changes, fostering collaboration and continuous delivery. This approach has improved my team's productivity by around 25% over the past year."
29. Can you discuss your experience with Continuous Integration/Continuous Deployment (CI/CD) pipelines?
Answer: "I have implemented CI/CD using tools like Jenkins. This setup automatically tests and deploys code changes, reducing the time from code approval to production deployment significantly."
30. How do you track your development progress?
Answer: "I use project management tools like Jira to organize tasks and track their progress. This transparency helps keep deadlines clear and responsibilities assigned."
Problem-Solving and Analytical Questions
31. How do you approach solving a complex problem?
Answer: "I decompose the problem into smaller parts and analyze each one. Using flowcharts helps me visualize dependencies and facilitates my understanding of the overall structure."
32. Can you share an example of a particularly challenging coding problem and how you solved it?
Answer: "I faced a challenge with a slow-running report query. After optimizing the SQL by adding indexes and rewriting filters, I improved the runtime from 7 seconds to under 1 second, enhancing user satisfaction."
33. What techniques do you use for effective troubleshooting?
Answer: "My systematic approach includes reproducing errors, checking logs for relevant data, and employing a debugger. Documenting past issues also helps streamline future troubleshooting."
34. How do you stay current with the latest software development trends?
Answer: "I follow respected tech blogs and participate in coding bootcamps. Engaging in online forums and contributing to open-source projects keeps my knowledge fresh and relevant."
35. Describe how you would handle conflicting requirements from stakeholders.
Answer: "I would organize a meeting to clearly understand all needs, prioritizing requirements based on project goals. Documenting everything helps manage expectations and find a consensus."
Soft Skills Questions
36. How do you communicate technical concepts to non-technical stakeholders?
Answer: "I focus on clear language, avoiding jargon. Using visual aids or analogies aids understanding. Explaining a new product feature to executives helped align our development with strategic goals."
37. Can you describe a situation where you took the initiative?
Answer: "I recognized inefficiencies in our deployment process. After researching CI/CD tools, I implemented a new pipeline, cutting deployment time by 40% and improving team morale."
38. What role do you typically take in team projects?
Answer: "I often act as a key collaborator, helping facilitate discussions and ensure inclusive input. I am also comfortable taking leadership roles when necessary to guide the team."
39. How do you manage stress during tight deadlines?
Answer: "I maintain focus through structured schedules and prioritize my workload. Practicing time management techniques reduces my anxiety, enabling me to perform effectively under pressure."
40. What are the most important skills for a software developer?
Answer: "Critical thinking, strong problem-solving abilities, and adept coding skills are essential. Additionally, effective communication and teamwork are vital for successful project outcomes."
Closing Questions
41. What do you know about Microsoft’s software development culture?
Answer: "Microsoft fosters innovation and collaboration, valuing diversity in thought and approach. The emphasis on inclusivity and work-life balance aligns with my own values."
42. Where do you see your career in five years?
Answer: "I see myself taking on a lead developer role with responsibilities in architectural decisions and mentoring. I aim to contribute significantly to impactful projects and innovate alongside a talented team."
43. What excites you most about working at Microsoft?
Answer: "Microsoft’s innovative culture and focus on impactful products truly excite me. The chance to work with advanced technologies and collaborate with skilled professionals suits my career aspirations."
44. Why should we hire you for this role?
Answer: "I bring a solid blend of technical skills, problem-solving abilities, and a collaborative approach. My diverse project experiences position me to adapt and thrive in dynamic environments."
45. What are your salary expectations?
Answer: "I seek a competitive salary reflecting my skills and experience. I believe we can discuss a fair agreement once we establish the project's context and demands."
Final Thoughts
Preparing for interviews is a transformative experience, especially at a dynamic company like Microsoft. While technical skills and experience matter greatly, showcasing your interpersonal attributes and passion for software development is equally vital.
This guide offers 50 interview questions and answers to structure your preparation, enabling you to demonstrate both competence and confidence during your interviews. Tailor your answers to reflect your unique experiences and proficiency to resonate with interviewers.
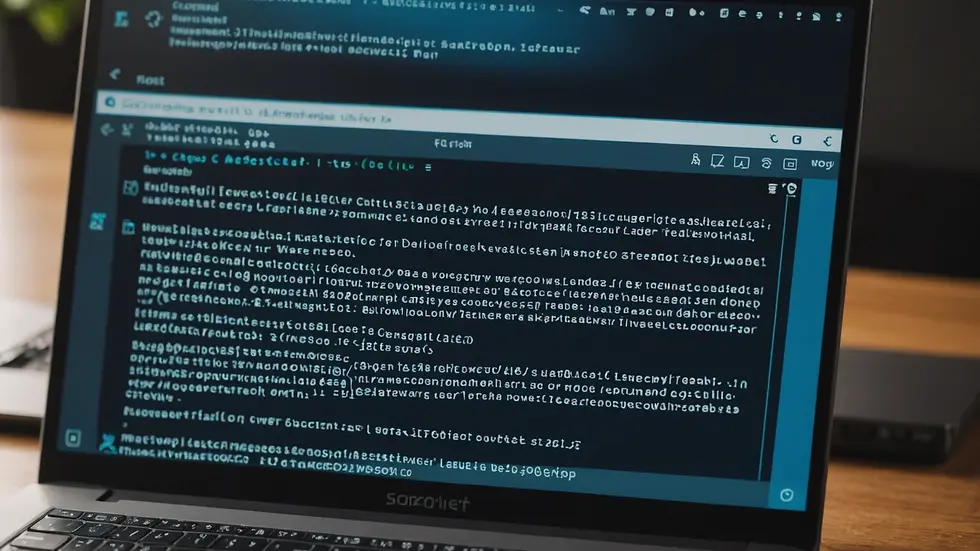
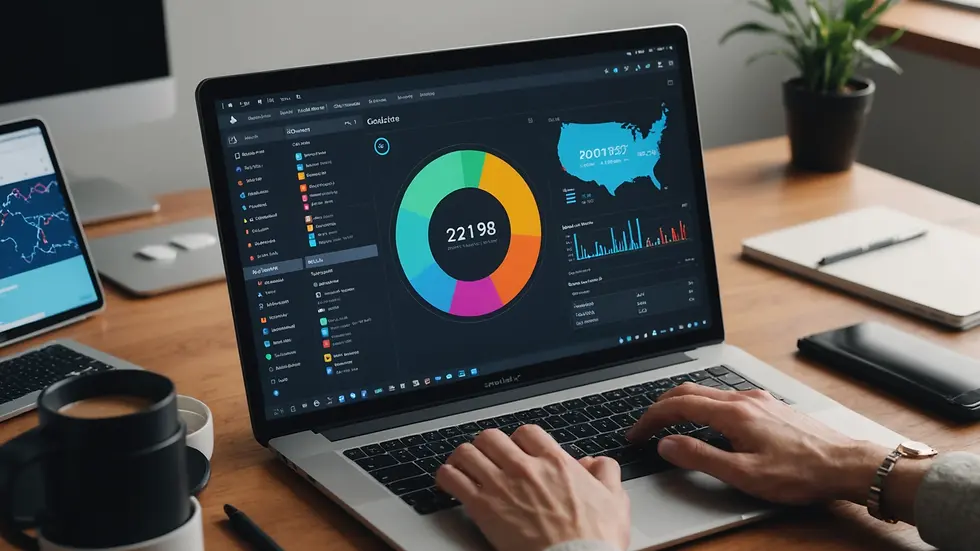
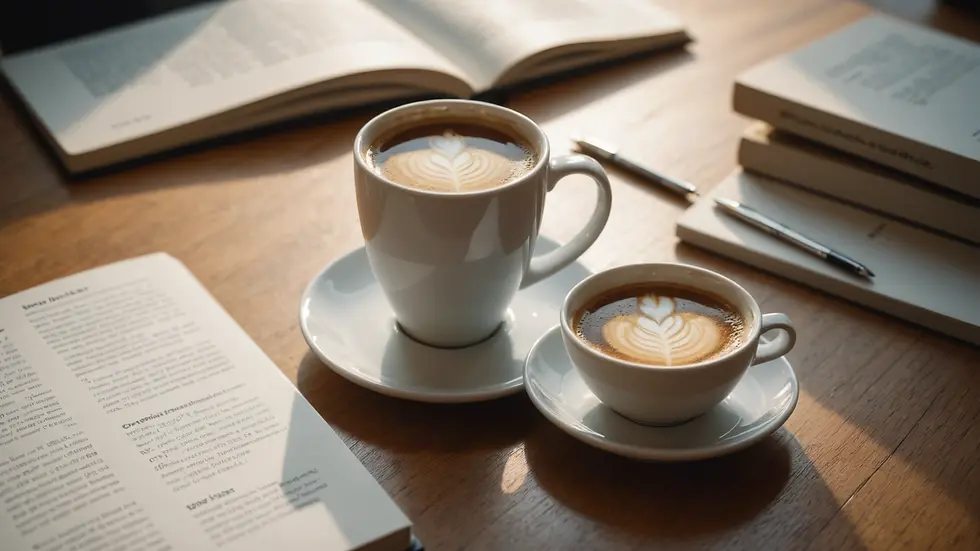
Embrace this chance to showcase your skills and knowledge. Good luck!