Interview Questions and Answers for Software Development at SAP
- Author
- Feb 11
- 7 min read
Navigating the interview process for a software development position at SAP requires not only a solid understanding of technical skills but also preparedness for behavioral and situational questions. Whether you are a seasoned developer or an entry-level candidate, understanding the types of questions you might face can significantly enhance your confidence and performance.
In this blog post, we will explore 50 interview questions and answers tailored for software development positions at SAP. Each question will be categorized into sections such as technical, behavioral, and situational, providing insights to help you prepare effectively.
Technical Questions
1. What programming languages are you proficient in?
Answer: I am proficient in Java, C#, and Python. I have utilized Java extensively for backend development and C# for building Windows applications. Python is my go-to language for data analysis and automation tasks.
2. Can you explain the concept of object-oriented programming (OOP)?
Answer: Object-oriented programming (OOP) is a programming paradigm that uses "objects" to represent data and methods. Key concepts include encapsulation, inheritance, polymorphism, and abstraction. These concepts help in designing modular and reusable code.
3. What is the difference between an abstract class and an interface?
Answer: An abstract class can have both abstract methods (without an implementation) and concrete methods (with an implementation), while an interface can only have abstract methods until Java 8, where default methods were introduced. A class can implement multiple interfaces but can inherit from only one abstract class.
4. Describe your experience with APIs.
Answer: I have designed and consumed RESTful APIs to connect various applications. My experience includes using tools like Postman to test API endpoints, handling authentication and data formats like JSON and XML, and ensuring the APIs are robust and scalable.
5. What is a data structure? Give examples.
Answer: A data structure organizes and stores data to enable efficient access and modification. Common examples include arrays, linked lists, stacks, queues, trees, and hash tables. Each has its own use cases depending on the problem at hand.
6. Explain the MVC architecture.
Answer: MVC stands for Model-View-Controller, a design pattern used to separate application logic into three interconnected components. The Model manages data and business logic, the View handles the user interface, and the Controller processes user input, updating the Model or View as necessary.
7. What experience do you have with database management systems?
Answer: I have experience with both SQL databases like MySQL and PostgreSQL, as well as NoSQL databases such as MongoDB. My responsibilities included designing schemas, writing queries, optimizing performance, and ensuring data integrity and security.
8. How do you handle version control in your projects?
Answer: I use Git for version control, allowing me to track changes to the codebase, collaborate with other developers, and manage branches effectively. I follow GitFlow for branching strategy and regularly commit changes with clear messages.
9. What is your approach to debugging?
Answer: My debugging process involves reproducing the error, isolating the problematic code, and using debugging tools to analyze the state of the application. I make use of logging and systematic testing to identify issues quickly.
10. Can you explain what Continuous Integration (CI) and Continuous Deployment (CD) are?
Answer: Continuous Integration (CI) is the practice of automatically testing code changes frequently and merging them into a shared repository. Continuous Deployment (CD) extends this practice by automatically deploying the validated code to production, ensuring a rapid release cycle.
Behavioral Questions
11. Describe a challenging project you worked on.
Answer: One challenging project involved developing a multi-tenant application that required complex user management features. I handled the design and implementation under tight deadlines, communicating effectively with team members to ensure timely delivery without compromising quality.
12. How do you prioritize tasks when working on multiple projects?
Answer: I prioritize tasks based on deadlines, business impact, and resource availability. I use tools like Trello for organizing tasks and ensure regular communication with stakeholders to adapt priorities as needed.
13. Describe a time you faced a conflict in a team setting.
Answer: In a previous project, team members had differing opinions on technology choices. I facilitated a meeting to discuss each option's merits and collectively evaluated the pros and cons. This approach helped the team reach a consensus and strengthened our collaboration.
14. How do you keep your skills updated?
Answer: I regularly participate in online courses, attend webinars, and follow industry blogs. Additionally, I engage in personal projects to apply new technologies, ensuring I remain competent in emerging trends.
15. Describe a situation where you had to adapt to a significant change.
Answer: In a recent project, we shifted from a Waterfall to an Agile development model. I quickly learned the Agile principles and adapted my work style accordingly, which helped the team enhance productivity and responsiveness to changes.
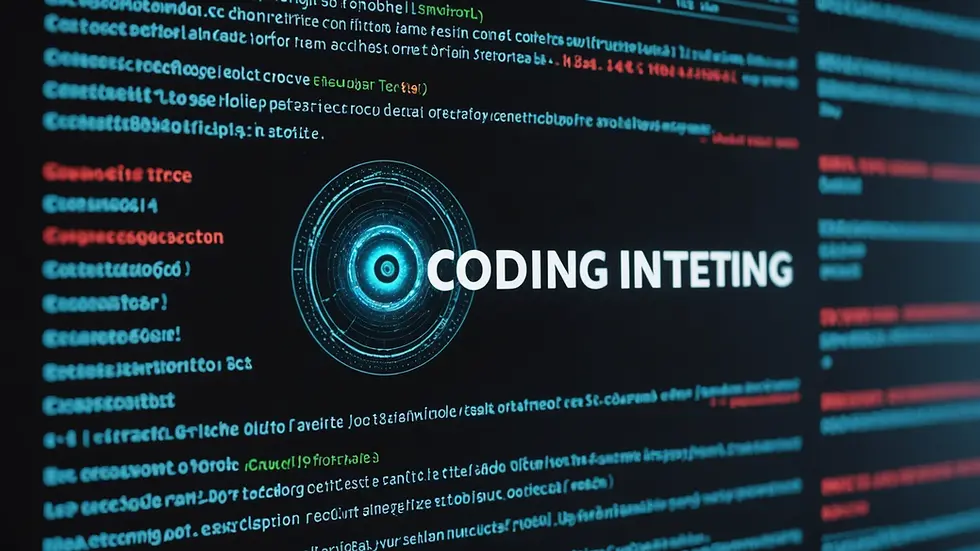
Situational Questions
16. What would you do if you realized you made a mistake in your code after it has been deployed?
Answer: I would immediately document the issue and inform my team lead, then work on a fix and prepare a rollback plan if necessary. Transparency is essential in minimizing the impact on users.
17. How would you deal with an underperforming team member?
Answer: I would have a one-on-one conversation to understand any challenges they might be facing and offer assistance. If the issue persists, I would involve relevant management to seek further support or training.
18. If you received critical feedback from a peer, how would you respond?
Answer: I would take time to reflect on the feedback and consider its validity. I believe that constructive criticism is an opportunity for growth, and I would seek further clarification if needed, applying suggestions to improve my performance.
19. How would you approach a project with unclear requirements?
Answer: I would schedule meetings with stakeholders to gather more information and clarify expectations. I would also advocate for the creation of a detailed requirement specification document to ensure everyone is aligned before moving forward.
20. What would you do if you were assigned a task that you believed was outside your skill set?
Answer: I would assess the situation and reach out for clarification on the task's requirements. If I felt unable to complete it, I would communicate this to my supervisor and express a willingness to learn, suggesting possible alternatives or requesting mentorship.
Advanced Technical Questions
21. What is multithreading and why is it important?
Answer: Multithreading is the concurrent execution of multiple threads in a single process, allowing better resource utilization and improved application performance. It is crucial in developing responsive applications and performing background tasks efficiently.
22. Can you explain the concept of garbage collection in Java?
Answer: Garbage collection is an automatic memory management feature in Java that removes objects that are no longer referenced in the program, freeing up memory. This process helps prevent memory leaks and reduces the overhead for developers regarding memory management.
23. How do you implement security measures in your applications?
Answer: I implement security measures by following best practices such as input validation, using encryption for sensitive data, implementing authentication and authorization protocols, and regularly conducting security audits.
24. What are design patterns, and can you give examples of commonly used ones?
Answer: Design patterns are reusable solutions to common design problems in software development. Examples include Singleton, Observer, Factory Method, and Strategy patterns. They help developers design applications that are easier to understand and maintain.
25. How do you ensure your code is maintainable?
Answer: I ensure code maintainability by following coding standards, writing documentation, conducting code reviews, and implementing unit tests. I also prioritize modular code structure to facilitate easy updates and modifications.
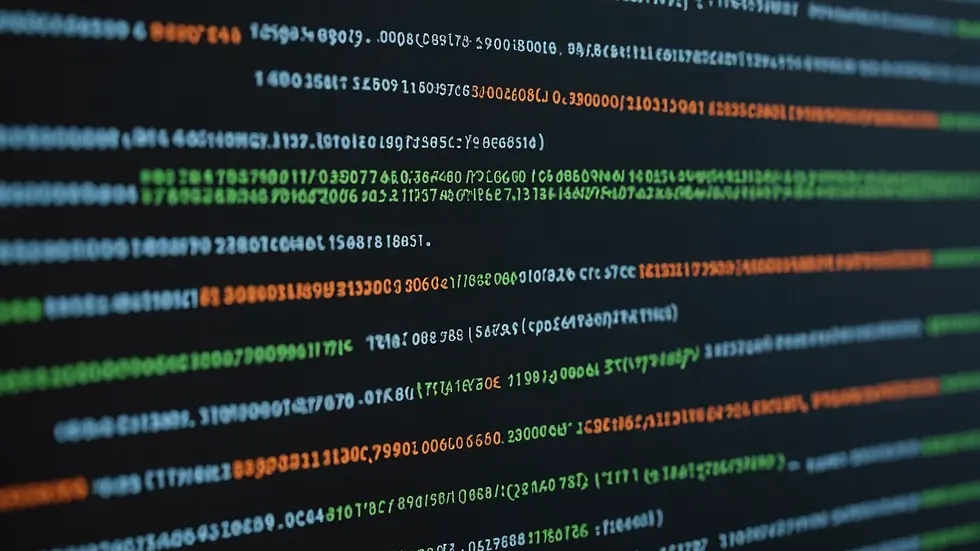
Behavioral and Situational Questions Continued
26. How do you handle tight deadlines?
Answer: I break down tasks into smaller, manageable pieces and prioritize them. If I foresee challenges in meeting deadlines, I communicate early with my team or manager to discuss potential solutions or adjustments.
27. Can you provide an example of how you worked in a diverse team?
Answer: I worked on a project with team members from various cultural backgrounds. I encouraged an inclusive atmosphere where everyone could voice opinions and contributed to a brainstorming session that ultimately enriched the project's outcomes.
28. How do you approach learning a new programming language?
Answer: I begin by understanding the language's syntax and core principles through online tutorials and documentation. Following that, I practice with small projects or exercises to cement my learning and apply concepts in real-world scenarios.
29. Describe a time when you went above and beyond in your job.
Answer: In a recent project, I volunteered to take on additional responsibilities, such as conducting peer reviews and sharing best practices. This commitment ensured that our team delivered a high-quality product while enhancing collaboration.
30. How do you ensure good communication with remote team members?
Answer: I utilize collaboration tools like Slack and video conferencing applications for regular updates and check-ins. I also make it a point to be clear and concise in my communications and encourage feedback to maintain an open dialogue.
Final Technical Questions
31. What are the differences between synchronous and asynchronous programming?
Answer: Synchronous programming executes tasks sequentially, blocking the program until the current task is finished. In contrast, asynchronous programming enables tasks to run concurrently, allowing the program to continue processing without waiting for each task to complete.
32. Can you explain the SOLID principles?
Answer: The SOLID principles are a set of design principles aimed at making systems more understandable and flexible. They include Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion principles.
33. What is a RESTful service, and how does it differ from a SOAP service?
Answer: A RESTful service uses HTTP requests for communication and is based on stateless operations, primarily using JSON. SOAP, on the other hand, is a protocol with stricter standards and relies on XML. REST is generally more lightweight and easier to integrate into web applications.
34. How do you approach testing in your development process?
Answer: I incorporate testing at every stage of development, utilizing unit tests for individual components and integration tests to ensure system components work together correctly. I also implement automated testing wherever possible to streamline the testing process.
35. What is continuous feedback and how do you utilize it?
Answer: Continuous feedback involves regularly gathering input from users and stakeholders throughout the development process. I implement it through frequent check-ins, beta testing phases, and user acceptance testing to refine the product based on real user behavior.
Conclusion
Preparing for an interview at SAP for a software development position can be a rigorous process, but thorough preparation can make a significant difference. By mastering both technical concepts and understanding the importance of behavioral and situational responses, candidates can showcase their skills effectively.
The questions and answers provided in this blog post cover a range of topics that can help you navigate your interview with confidence. Remember to approach each question thoughtfully, providing examples from your experience that demonstrate your knowledge, problem-solving abilities, and teamwork skills.
By diligently preparing for your interview with these 50 interview questions and answers, you position yourself as a strong candidate ready to contribute to SAP's innovative projects and initiatives.
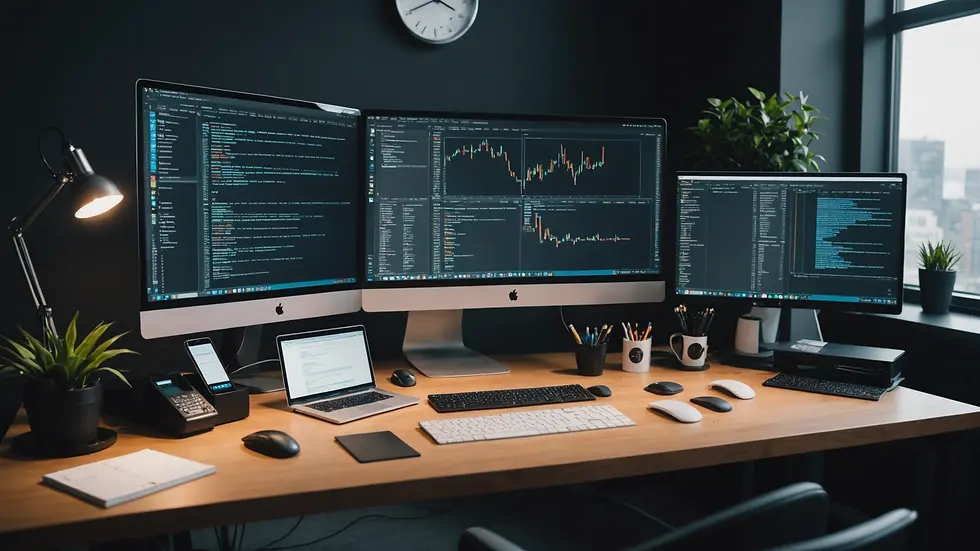