The path to becoming a software engineer often comes with excitement and unpredictability, especially during interviews. These interviews can act as both a challenge and an opportunity, determining who secures that sought-after role. It is essential for candidates to prepare well to navigate this demanding process. In this blog post, we will explore 50 interview questions and answers specifically designed for software engineers.
These questions span from technical skills to soft skills and behavioral inquiries, giving you a well-rounded understanding of what to expect during software development interviews.
Technical Questions
1. What is a data structure, and why are they important?
A data structure is a specific way of organizing and storing data so that it can be accessed and modified efficiently. For instance, using a hash table can improve the time complexity of search operations to O(1) on average. In contrast, using an unordered list may require O(n) time. Given that software engineers often deal with large datasets, understanding data structures is critical for optimizing performance.
2. Explain the difference between an array and a linked list.
An array is a static data structure that holds a fixed-size collection of elements, allowing quick access through indices. For example, accessing an element in an array takes O(1) time. On the other hand, a linked list is a dynamic data structure made up of nodes. Each node has data and a pointer to the next node, enabling easier insertions and deletions without needing to resize. However, accessing an element in a linked list requires O(n) time.
3. What is the time complexity of a binary search algorithm?
The time complexity of a binary search algorithm is O(log n), where `n` is the number of elements in a sorted array. For instance, if you have an array of 1,024 elements, a binary search can find an element in no more than 10 comparisons. This efficiency makes binary search a preferred method for searching sorted data.
4. Can you explain what a hash table is?
A hash table is a data structure that maps keys to values using a hash function. The hash function calculates an index within an array, allowing for quick data retrieval. For example, correctly designed hash tables can offer average-case time complexities of O(1) for insertions, deletions, and lookups.
5. What are the various types of sorting algorithms?
Sorting algorithms can be divided into two main categories: comparison sorts and non-comparison sorts. Comparison sorts, like Quick Sort and Merge Sort, typically have time complexities of O(n log n). Non-comparison sorts, such as Counting Sort, can sort in O(n) time but require special conditions, such as limited range of integer keys.
6. Explain the concept of object-oriented programming (OOP).
Object-oriented programming (OOP) is a programming paradigm that uses "objects" to represent data and methods. Key principles of OOP include:
Encapsulation: Bundling data and methods that operate on that data within one unit, limiting access to the inner workings.
Inheritance: Allowing new classes to inherit properties and behavior from existing classes.
Polymorphism: Enabling methods to do different things based on the object calling them.
For example, in a banking application, a `SavingsAccount` class can inherit properties from a `BankAccount` class but also have unique behaviors.
7. What is a RESTful API?
A RESTful API is an interface that uses HTTP requests to manage data. It follows principles of Representational State Transfer (REST), making it stateless. For example, it can use GET to retrieve data, POST to create data, PUT to update, and DELETE to remove data. Many web services use RESTful APIs, like Twitter's and GitHub’s, to allow developers to interact with their platforms.
8. Describe the Model-View-Controller (MVC) architecture.
The MVC architecture separates an application into three parts:
Model: Represents the data and business logic.
View: The user interface that presents data to the user.
Controller: The logic that interacts with the model and the view.
This separation allows for better management of code and easier modifications. Many frameworks, like Ruby on Rails and ASP.NET MVC, leverage this pattern for web applications.
9. What is the difference between synchronous and asynchronous programming?
Synchronous programming executes tasks one after another, requiring each task to complete before starting the next. In contrast, asynchronous programming allows tasks to execute separately, enabling the program to continue running while waiting for a resource (like a database response). For example, a web server can handle multiple requests simultaneously using asynchronous patterns, improving performance significantly.
10. Can you explain SQL and NoSQL databases?
SQL databases are structured and use structured query language for operations. For example, databases like MySQL and PostgreSQL are designed for complex queries and relationships. On the other hand, NoSQL databases, such as MongoDB and Cassandra, provide more flexibility in data types and structures, making them suitable for scenarios like handling big data or unstructured data.
Behavioral Questions
11. Describe a challenging technical problem you faced and how you solved it.
When you discuss a challenging problem, use a specific situation. For example, explain how you encountered performance issues in a web application and what you did to resolve it. Perhaps you optimized the database queries, reducing response time by 30%.
12. How do you prioritize tasks when working on multiple projects?
Share your approach to prioritizing tasks. For example, you might use tools like Trello or Asana to assess deadlines based on urgency and importance. Highlight techniques for effective time management, such as breaking projects into smaller tasks or using the Eisenhower Box method to categorize tasks.
13. Tell me about a time when you received constructive criticism.
When answering, use the STAR method (Situation, Task, Action, Result). Describe a real situation where you received feedback, how you applied it, and the beneficial outcome that followed. For instance, explain how feedback improved your code quality and increased team efficiency.
14. How do you keep current with new technologies and programming languages?
Discuss the resources you use to stay informed, such as online platforms like Udemy, Coursera, or tech communities like Stack Overflow. Mention your participation in webinars or meetups to engage with experts and share knowledge. This approach illustrates your dedication to ongoing learning.
15. Describe your experience working in a team environment.
Provide an example of a team project you contributed to. Discuss your role, how you communicated with team members, and the value of collaboration in achieving the project's goals. Perhaps mention a project where effective teamwork led to a successful product release ahead of schedule.
16. How do you handle tight deadlines?
Talk about your techniques for managing stress. You can mention methods such as breaking projects into manageable tasks or maintaining open communication with teammates. Emphasize your commitment to delivering quality work, even under pressure.
17. What motivates you to do your best work?
Share what drives your passion for software engineering. This might include the satisfaction of problem-solving, creating innovative solutions, or the excitement of learning new technologies. Authentic motivation reflects positively during interviews.
18. How do you handle conflict in a team?
Describe your conflict resolution strategy, underscoring the importance of communication. Provide examples of when you brought team members together to address differing opinions focused on achieving a common goal.
19. What is your greatest strength as a software engineer?
Identify a key strength relevant to the role, such as problem-solving skills or adaptability. Provide specific examples from your work experience where this strength benefited a project.
20. What is your greatest weakness as a software engineer?
Select a genuine weakness and discuss how you are working to improve it. For instance, if you tend to overanalyze code, explain how you’ve sought mentorship or set deadlines to improve efficiency without sacrificing quality.
Strategic Questions
21. What programming languages are you proficient in?
List the programming languages you know, mentioning projects where you applied them. For code like Java, Python, or JavaScript, state specific frameworks you have experience with, such as Spring or React.
22. Describe a project you're most proud of.
Select a project that highlights your skills and the technologies you used. Explain the problem it solved, its impact, and the valuable lessons learned from the experience.
23. How do you ensure the quality of your code?
Discuss practices you implement for maintaining high-code quality, such as doing thorough code reviews, employing rigorous testing methods, and utilizing version control. Explain how finding bugs before deployment minimizes potential issues.
24. What is automated testing, and why is it important?
Automated testing involves using software to execute tests on your code automatically. It enhances efficiency by allowing frequent tests as code changes occur. Studies show that teams using automated testing report a 30% decrease in bugs post-release.
25. How do you approach debugging a complex issue?
Explain your debugging process, including isolating the problem, using logs for insights, and collaborating with team members when necessary. This clarity can show your methodical approach, improving the chances of resolution.
26. How do you handle code reviews?
Describe your positive and constructive perspective on code reviews, highlighting their role in improving code quality. Share how you provide and accept feedback, viewing it as an opportunity for growth.
27. What version control systems have you used?
Mention specific tools such as Git or SVN, highlighting your familiarity and experience with particular features, like branching or merging. Discuss any team workflows you've worked with.
28. Describe your experience with agile methodologies.
Share your involvement with agile practices, such as participating in sprints, stand-ups, or retrospectives. Explain your role in these methodologies, such as being a Scrum Master or a contributor within a team.
29. How do you deal with technical debt?
Address your understanding of technical debt and offer strategies for managing it, like regular refactoring or incorporating tech debt reviews during development cycles. Emphasizing proactive management shows responsibility in maintaining code health.
30. What are design patterns, and can you give an example?
Design patterns are standard solutions to common software design problems. Examples include the Singleton pattern for managing shared resources, and the Observer pattern for event-driven systems. Be prepared to discuss scenarios in which you've used these patterns effectively.
Problem-Solving Questions
31. Write a function to reverse a string.
```python
def reverse_string(s):
return s[::-1]
```
32. Explain how you would implement a stack using queues.
You can implement a stack using two queues. Push an item into one queue and during a pop operation, move items to the second queue to reverse their order.
33. How would you find the largest element in an unsorted array?
You would iterate through the array, maintaining a variable that stores the maximum value. This approach operates in O(n) time complexity, ensuring you're efficient.
34. Can you describe what a binary tree is?
A binary tree is a data structure where each node has at most two children, known as the left child and the right child. It facilitates various operations like searching, insertion, and deletion efficiently.
35. How would you search a linked list?
You would traverse through the linked list, checking each node's value until you find the target. This operation typically has a time complexity of O(n), which makes searching through large datasets more challenging.
36. Explain the difference between depth-first search (DFS) and breadth-first search (BFS).
DFS goes deep down one branch before backtracking, while BFS explores all neighbors at the present depth before proceeding to the next level. Graph traversal algorithms benefit significantly from both methods, depending on the use case.
37. Describe how to detect a cycle in a linked list.
Utilize Floyd’s cycle detection algorithm, which employs two pointers moving at different speeds. If the two pointers meet at some point, it indicates a cycle exists in the list.
38. Write a function to remove duplicates from an array.
```python
def remove_duplicates(arr):
return list(set(arr))
```
39. Explain what a deadlock is and how to prevent it.
A deadlock occurs when two or more processes wait indefinitely for each other to release resources. You can prevent deadlocks by using methods like resource allocation graphs, assigning priorities to resource requests, or avoiding circular waiting scenarios.
40. Describe a system you designed from scratch.
Discuss a system you built, focusing on the problem it solved, technologies you used, and your thought process during its design. Highlight any specific challenges you faced and how you overcame them.
Final Remarks
Preparing for a software engineering interview may seem daunting, but being familiar with common questions and their answers can make a significant impact. Throughout this post, we have explored 50 essential interview questions and answers ranging from technical fundamentals to behavioral assessments.
Aspiring software engineers should use this guide not only to demonstrate their knowledge but also to show their growth mindset, collaborative spirit, and dedication to excellence.
Keep this guide close as you prepare for interviews. Best of luck landing your dream position as a software engineer!
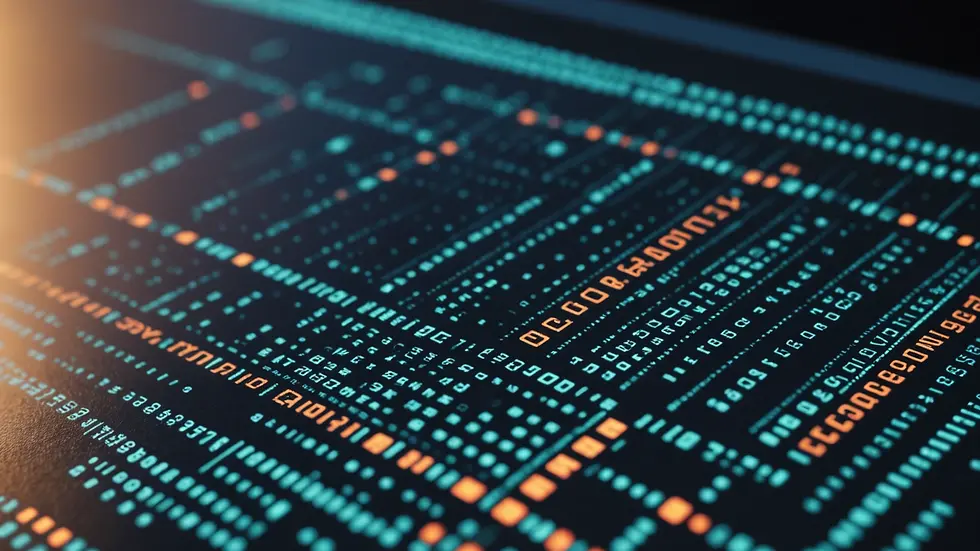