Interview Questions and Expert Answers for Software Development at Nvidia
- Author
- Feb 11
- 7 min read
Navigating the competitive landscape of software development interviews at Nvidia can be challenging yet rewarding. Candidates often seek insight into the types of questions they may encounter during the interview process. This blog post aims to present a comprehensive list of 100 interview questions along with expert answers that can help you prepare for your interview at Nvidia.
Understanding Nvidia and Its Interview Process
Nvidia is renowned for its contributions to the field of graphics processing, artificial intelligence, and high-performance computing. The company's reputation attracts many developers, making the selection process highly competitive. Understanding the interview structure and the nature of questions asked can significantly enhance your preparation.
Nvidia typically focuses on technical skills, problem-solving abilities, and cultural fit during interviews. Candidates may encounter coding challenges, theoretical questions, and situational or behavioral questions.
Technical Questions
1. What is the difference between a process and a thread?
A process is an independent program that runs in its own memory space, while a thread is a smaller unit of execution within a process that shares the same memory space with other threads. Threads can communicate more easily than processes due to shared memory.
2. Explain the concept of memory leaks.
A memory leak occurs when a program allocates memory but fails to release it back to the operating system after use. Over time, this can lead to decreased performance and application crashes as available memory is exhausted.
3. Describe polymorphism in object-oriented programming.
Polymorphism allows methods to do different things based on the object it is acting upon. There are two types: compile-time (method overloading) and runtime (method overriding). This promotes versatility and flexibility in programming.
4. What are the basic principles of SOLID?
SOLID is an acronym for five design principles that make software designs more understandable, flexible, and maintainable. It includes:
S: Single Responsibility Principle
O: Open/Closed Principle
L: Liskov Substitution Principle
I: Interface Segregation Principle
D: Dependency Inversion Principle
5. What is a deadlock? How can it be avoided?
A deadlock occurs when two or more processes are unable to proceed because each is waiting for the other to release a resource. Deadlocks can be avoided by following a proper resource allocation strategy, such as using a lock hierarchy or implementing timeout strategies.
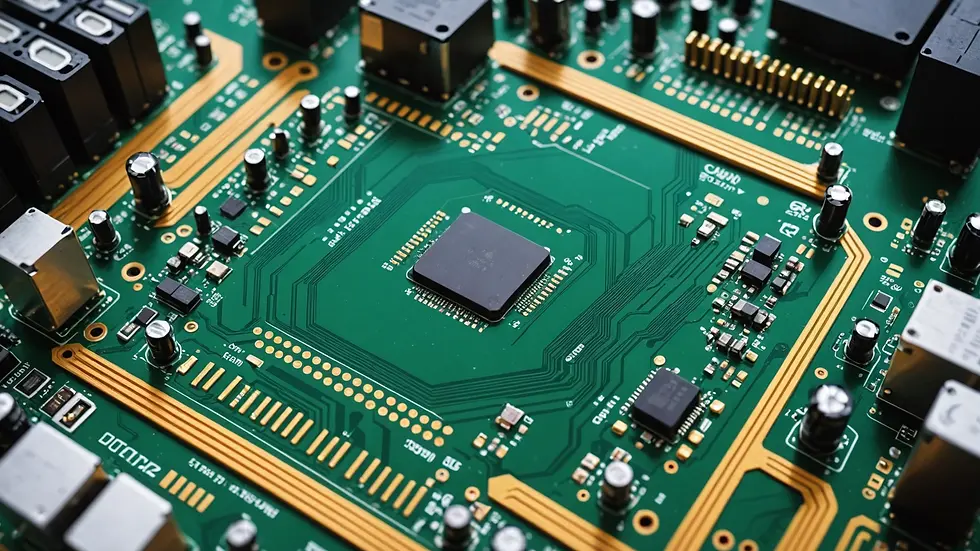
6. What is the Big O notation, and why is it important?
Big O notation is a mathematical representation that describes the performance or complexity of an algorithm in terms of time or space. It is crucial for evaluating the efficiency of algorithms and comparing their performance.
7. Differentiate between synchronous and asynchronous programming.
Synchronous programming executes tasks in a sequential manner, where each task must complete before the next one starts. Asynchronous programming allows tasks to run independently, enabling the execution of other tasks without waiting for one to finish, improving performance in I/O-bound applications.
8. Explain what a hash table is.
A hash table is a data structure that stores key-value pairs, allowing for fast data retrieval. It uses a hash function to compute an index where the value is stored. This results in an average time complexity of O(1) for lookups.
9. What is a binary tree, and how does it differ from a binary search tree?
A binary tree is a tree data structure where each node has at most two children. A binary search tree is a special type of binary tree where the left subtree contains nodes with values less than the parent node, and the right subtree has nodes with values greater.
10. Describe the concept of RESTful APIs.
REST (Representational State Transfer) is an architectural style for designing networked applications that rely on stateless communication and use standard HTTP methods. RESTful APIs are built around resources that can be created, read, updated, or deleted (CRUD) and can respond with data in JSON or XML format.
Behavioral Questions
11. Describe a challenging technical problem you faced and how you solved it.
In a previous project, I encountered a performance issue with a data processing pipeline. After analyzing the code, I identified a bottleneck that was caused by inefficient algorithms. I restructured the code and implemented a more efficient data handling strategy, which significantly improved performance.
12. How do you handle tight deadlines?
I prioritize tasks by assessing their impact and urgency. Effective communication with my team is crucial, so everyone is aligned on deadlines and expectations. I also use time management techniques to ensure high productivity under pressure.
13. Tell us about a time you worked in a team. What was your role?
In my last project, I served as the lead developer in a team responsible for a mobile application. I coordinated between designers and developers, ensuring that everyone understood the requirements, while also contributing code for critical features.
14. How do you approach debugging?
I start by replicating the issue to better understand its nature. Using debugging tools, I analyze logs and identify the root cause. After pinpointing the problem, I implement and test a solution to confirm it resolves the issue without introducing new bugs.
15. Describe a scenario where you had to learn a new technology quickly.
During a project transition, I had to adopt a new framework that was unfamiliar to me. I dedicated time to study its documentation, watched tutorials, and worked on small prototypes to gain practical experience. This proactive approach allowed me to contribute effectively in no time.
System Design Questions
16. How would you design a URL shortening service like bit.ly?
I would create a web application with the following components:
Frontend: A simple user interface for users to input and receive shortened URLs.
Backend: A server that generates a unique identifier for each URL and stores it in a database along with the original URL.
Database: A relational database to store original URLs and their shortened identifiers.
Redirect Service: When a user visits a shortened URL, the service queries the database and redirects them to the corresponding original URL.
17. What architecture would you use for a real-time chat application?
I would choose a microservices architecture with components including:
User Authentication Service: Manages user sessions and authentication.
Chat Service: Handles real-time messaging, possibly using WebSockets for instant delivery.
Storage Service: For persisting chat history and user data.
Load Balancer: To distribute incoming requests and ensure scalability.
18. Describe how you would design a file storage service like Dropbox.
The file storage service would consist of:
User Interface: Allowing users to manage files effortlessly.
API: Providing access to file upload, delete, and share functionalities.
Data Storage: Using a distributed file system or cloud storage to ensure redundancy and availability.
Synchronization Mechanism: To keep files updated across devices.
Authentication Service: To secure user data and permissions.
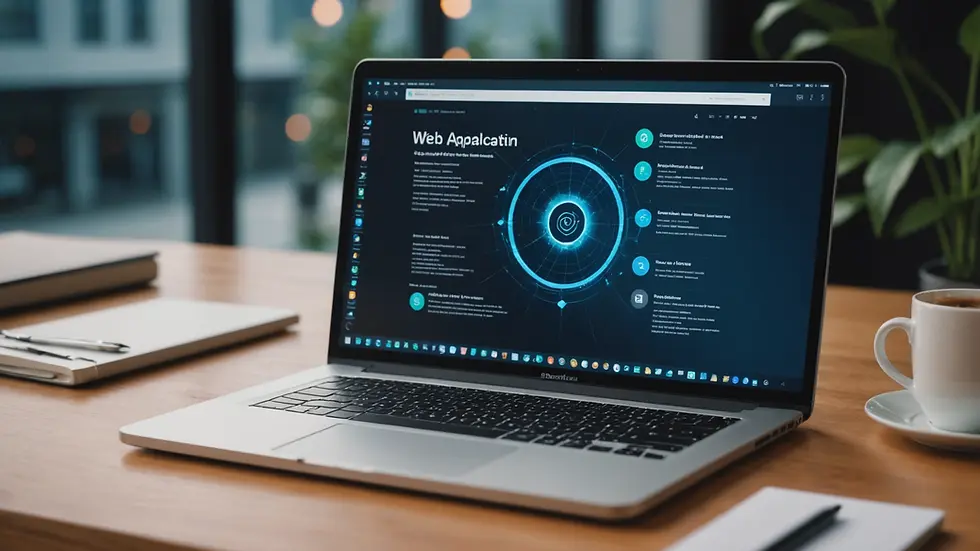
19. How would you implement caching in your application?
I would use caching at various layers:
Database Cache: Save results of frequent queries using an in-memory store (like Redis or Memcached) to reduce database load.
Application Cache: Store commonly accessed data temporarily in memory to enhance response times.
CDN: Employ a Content Delivery Network to cache static assets closer to the user.
20. What are some strategies to ensure high availability in web applications?
To ensure high availability, I would implement:
Load Balancing: Distributing traffic across multiple servers to prevent single points of failure.
Clustering: Running multiple instances of services to provide redundancy.
Regular Backups: Keeping up-to-date backups to restore service in case of data loss.
Monitoring: Continuous logging and monitoring to catch and respond to issues swiftly.
Coding Questions
21. Write a function to reverse a string.
```python
def reverse_string(s):
return s[::-1]
```
This simple Python function uses slicing to reverse the input string efficiently.
22. How would you find the longest substring without repeating characters?
```python
def longest_substring(s):
char_set = set()
left, max_length = 0, 0
for right in range(len(s)):
while s[right] in char_set:
char_set.remove(s[left])
left += 1
char_set.add(s[right])
max_length = max(max_length, right - left + 1)
return max_length
```
This function uses a sliding window approach to track characters and lengths.
23. How do you sort an array of integers?
```python
def sort_array(arr):
return sorted(arr)
```
Utilizing Python’s built-in sorting function ensures optimal performance and readability.
24. Write a function to check for balanced parentheses.
```python
def is_balanced(s):
stack = []
mapping = {')': '(', '}': '{', ']': '['}
for char in s:
if char in mapping:
top_element = stack.pop() if stack else '#'
if mapping[char] != top_element:
return False
else:
stack.append(char)
return not stack
```
This function efficiently tracks balance using a stack data structure.
25. How would you remove duplicates from a linked list?
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def remove_duplicates(head):
current = head
seen = set()
prev = None
while current:
if current.val in seen:
prev.next = current.next
else:
seen.add(current.val)
prev = current
current = current.next
return head
```
This approach utilizes a set for quick lookups to avoid duplicates.
Advanced Questions
26. Explain the CAP theorem.
The CAP theorem states that in a distributed data store, it is impossible to simultaneously guarantee all three of the following properties:
Consistency: All nodes in the cluster see the same data at the same time.
Availability: Every request receives a response, regardless of the state of the system.
Partition Tolerance: The system continues to operate despite network partitions.
27. What strategies would you use to optimize a SQL query?
To optimize SQL queries, I would:
Use indexes: Create appropriate indexes on frequently queried columns.
Avoid SELECT *: Only retrieve necessary columns to reduce data load.
Utilize joins efficiently: Ensure that joins are based on indexed columns.
Analyze execution plans: Review query execution plans to identify bottlenecks.
28. How do you ensure code quality in your projects?
I ensure code quality through:
Code reviews: Encouraging peer reviews to catch issues early.
Automated testing: Using unit tests and integration tests to validate functionalities.
Coding standards: Adopting consistent coding styles to improve readability and maintainability.
29. What is microservices architecture? What are its benefits?
Microservices architecture is a software design pattern in which applications are composed of small, independent services that communicate with each other through APIs. Benefits include:
Scalability: Each service can be scaled independently according to its needs.
Flexibility: Developers can use different technologies for different services.
Resilience: Failure in one service does not impact the entire system.
30. How can you implement logging in a large application?
Implement logging by using a centralized logging framework that supports various log levels (INFO, DEBUG, ERROR) and can aggregate logs from different services. Consideration for log rotation and archiving is essential to manage log size effectively.
Conclusion
Preparing for software development interviews at Nvidia can be an exhilarating journey when you approach it with the right tools and knowledge. By understanding common technical questions, behavioral queries, system design, and coding challenges, candidates can enhance their performance significantly.
Conducting thorough practice, reviewing these 100 interview questions and expert answers, and focusing on personal experiences will greatly aid in making a lasting impression during your Nvidia interview journey. Remember, the key to success lies not only in knowing the answers but also in demonstrating problem-solving skills and effective communication.
---
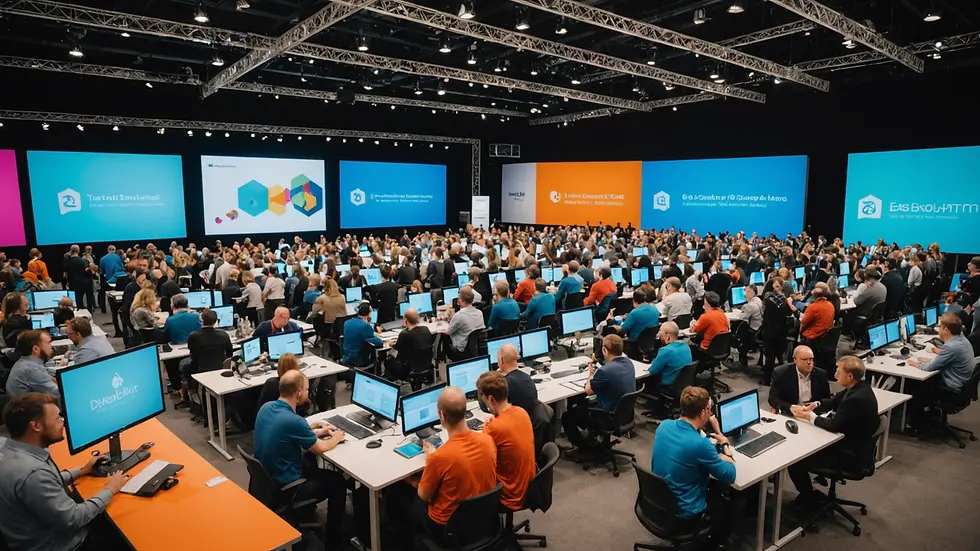